In this C++ tutorial, you will learn how to check if the given string contains only digits, with example programs.
Check if the string contains only digits in C++
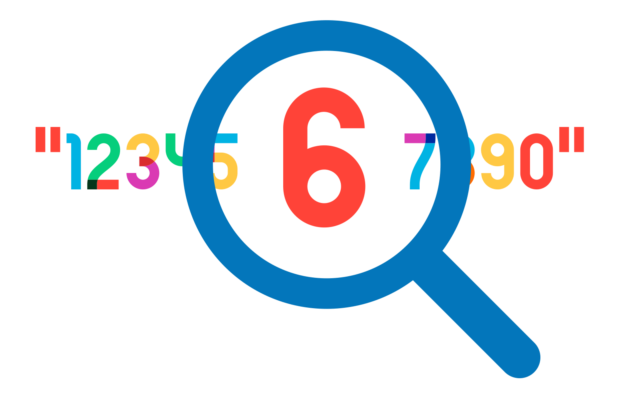
To check if the given string contains a specific substring in C++, you can use any of the following approaches.
- Using all_of algorithm with isdigit() function.
- Writing a For loop that iterates through characters in string, and check if each of the character is a digit using isdigit() function.
- Using regular expression function like regex_match() to check if the given string matches only digits.
1. Checking if string contains only digits using all_of algorithm and isdigit() method in C++
In the following program, we are given a string in str. We have to check if the string str contains only digits using all_of() function of algorithm library, and isdigit() method.
Steps
- Given an input string in
str
. - Call
all_of()
function from algorithm library. Pass string beginning and string ending as first and second arguments respectively. This lets all_of() to search from the beginning of the string to the ending of the string. Pass::isdigit
as third argument. This lets all_of() method to apply the isdigit() method for each of the character in the string. all_of()
returns a boolean value. Store it inallDigitsOnly
.- Use this boolean value
allDigitsOnly
as a condition in C++ if else statement. If-block executes when string contains only digits. Else-block executes when all the characters in the string are not digits.
Please note that you may have to check if the string is not empty before checking the string for digits.
Program
main.cpp
#include <iostream> #include <string> #include <algorithm> // For all_of function using namespace std; int main() { string str = "123"; bool allDigitsOnly = all_of(str.begin(), str.end(), ::isdigit); if (str != ""){ if (allDigitsOnly) { cout << "The string contains only digits." << endl; } else { cout << "The string contains non-digit characters." << endl; } } else { cout << "Empty string. Please check." << endl; } return 0; }
Output
The string contains only digits.
Now, let us change the value in str such that the string contains some alphabets.
main.cpp
#include <iostream> #include <string> #include <algorithm> // For all_of function using namespace std; int main() { string str = "Abc123"; bool allDigitsOnly = all_of(str.begin(), str.end(), ::isdigit); if (str != ""){ if (allDigitsOnly) { cout << "The string contains only digits." << endl; } else { cout << "The string contains non-digit characters." << endl; } } else { cout << "Empty string. Please check." << endl; } return 0; }
Output
The string contains non-digit characters.
2. Checking if string contains only digits using For loop and isdigit() method in C++
In the following program, we are given a string in str. We have to check if the string str contains only digits using For loop and isdigit() method.
Steps
- In the main() function, given an input string in
str
. - Define a function
containsOnlyDigits()
that takes a string as argument, and returns a boolean value of true if the string contains only digits, or false otherwise. - Inside the
containsOnlyDigits()
function, write a C++ For each loop to iterate through the characters in the string.- For each character
ch
in the string, check if the character is a digit using isdigit() function. Call isdigit() and pass the characterch
as argument. If the character is a digit, it returns true, otherwise returns false. - If the character is not a digit, return false.
- For each character
- After the For loop, when all the characters in the string
str
are checked out to be digits, return true. - Back to the main() function, we can call the
containsOnlyDigits()
function with the given stringstr
passed as argument, and use the returned value as a condition in C++ if else statement, where if-block executes if the string contains only digits, and else-block executes otherwise.
Please note that the containsOnlyDigits()
function returns true for a given empty string.
Program
main.cpp
#include <iostream> #include <string> #include <cctype> // For isdigit function using namespace std; bool containsOnlyDigits(const string& str) { for (char c : str) { if (!isdigit(c)) { return false; // If a non-digit character is found, return false } } return true; // If all characters are digits, return true } int main() { string str = "123"; if (containsOnlyDigits(str)) { cout << "The string contains only digits." << endl; } else { cout << "The string contains non-digit characters." << endl; } return 0; }
Output
The string contains only digits.
3. Checking if string contains only digits using regex_match() method in C++
In the following program, we are given a string in str. We have to check if the string str contains only digits using regex_match() function of regex library.
Steps
- Given an input string in
str
. - Call
regex_match()
function from regex library. Pass the stringstr
and the regular expressionregex("\\d+")
to match one or more digits. regex_match()
returns a boolean value. Store it inallDigitsOnly
.- Use this boolean value
allDigitsOnly
as a condition in C++ if else statement. If-block executes when string contains only digits. Else-block executes when all the characters in the string are not digits.
Please note that you may have to check if the string is not empty before checking the string for digits.
Program
main.cpp
#include <iostream> #include <string> #include <regex> // For regex_match function using namespace std; int main() { string str = "123"; bool allDigitsOnly = regex_match(str, regex("\\d+")); if (str != ""){ if (allDigitsOnly) { cout << "The string contains only digits." << endl; } else { cout << "The string contains non-digit characters." << endl; } } else { cout << "Empty string. Please check." << endl; } return 0; }
Output
The string contains only digits.
Conclusion
In this C++ Tutorial, we learned how to check if the string contains only digits through various approaches, with examples.