In this C++ tutorial, you will learn how to check if a given string contains a specific character, with example programs.
Check if the string contains specific character in C++
To check if the given string contains a specific character in C++, you can use find() method of string class. Or you can iterate over the characters in the string, and check if the character in the string equals the specified character.
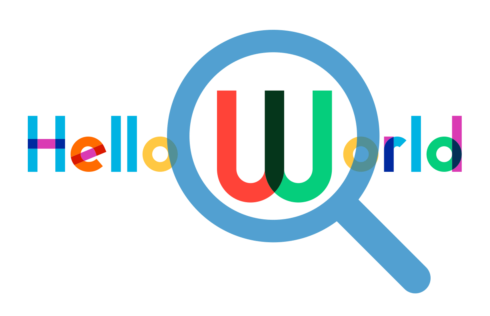
1. Checking if string contains character using find() method in C++
In the following program, we are given a string in str and the character in ch. We have to check if the string str contains the character ch using find() method.
Steps
- Given an input string in
str
, and a specific character inch
. - Call the
find()
method of thestring
class to search for the characterch
within thestr
. - If the
find()
method returns a value other thanstring::npos
, it means the specified character was found, indicating thatstr
contains the characterch
. Use this information to create a condition that returns true if the stringstr
contains the characterch
. - Write a C++ if else statement with the condition created from the previous step.
Program
main.cpp
#include <iostream> #include <string> using namespace std; int main() { string str = "Hello World"; char ch = 'W'; if (str.find(ch) != string::npos) { cout << "The string contains the character." << endl; } else { cout << "The string does not contain the character." << endl; } return 0; }
Output
The string contains the character.
Now, let us change the character ch value such that the string str does not contain the specified character, and run the program again.
main.cpp
#include <iostream> #include <string> using namespace std; int main() { string str = "Hello World"; char ch = 'P'; if (str.find(ch) != string::npos) { cout << "The string contains the character." << endl; } else { cout << "The string does not contain the character." << endl; } return 0; }
Output
The string does not contain the character.
2. Checking if string contains character using For loop in C++
In the following program, we have to check if the string str contains the character ch using C++ For loop.
Steps
- Given an input string in
str
, and a specific character inch
. - Take a boolean variable
isCharFound
to store the information that if the character is found or not. - Write a For loop to iterate over the characters in the string
str
. Inside the For loop if the character in the string matches the specified characterch
, setisCharFound
to true, and break the loop. After the completion of For loop,isCharFound
is set to true if specified characterch
is in the stringstr
, or else false. - Write a C++ if else statement with
isCharFound
value as condition and print to output whether the character is present in the string or not.
Program
main.cpp
#include <iostream> #include <string> using namespace std; int main() { string str = "Hello World"; char ch = 'P'; bool isCharFound = false; for (char c : str) { if (c == ch) { isCharFound = true; break; } } if (isCharFound) { cout << "The string contains the character." << endl; } else { cout << "The string does not contain the character." << endl; } return 0; }
Output
The string contains the character.
Conclusion
In this C++ Tutorial, we learned how to check if the string contains the specified character using string find() method, or For loop, with examples.