In this C++ tutorial, you will learn how to check if a given string starts with a specific prefix value, with example programs.
Check if the string starts with a specific prefix in C++
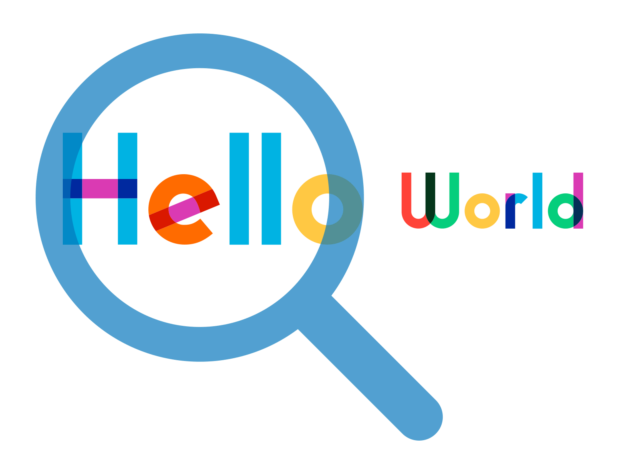
To check if the string starts with a specific prefix value in C++, get the substring of the given string that starts at index=0 and spans a length as that of the specified prefix value, and then compare it to the specified prefix value.
If the substring equals the prefix value, then we can say that the given string starts with the specified prefix value. Otherwise the given string does not start with the specified value.
1. Checking if the string starts with a specific prefix using substring and string equality in C++
In the following program, we take a string in str
and a prefix string value in prefix
. We have to check if the string str
starts with the prefix string prefix
using string substr() method and string equality check.
Steps
- Given an input string in
str
, and a prefix string inprefix
. - Call the
substr()
method of thestring
class to extract a substring ofstr
starting from index 0 and having a length equal to the length ofprefix
. Store the extracted substring in variablesubstr
. - Then compare the substring with the
prefix
string using the==
operator. - If the substring equals the prefix, then the string
str
starts with the specified prefix value. Else the stringstr
does not start with the specified prefix value. You can use C++ If Else statement to implement this step, withsubstr == prefix
as the if-condition.
Program
main.cpp
#include <iostream> #include <string> using namespace std; int main() { string str = "Hello World"; string prefix = "Hello"; if (str.substr(0, prefix.length()) == prefix) { cout << "The string starts with the prefix." << endl; } else { cout << "The string does not start with the prefix." << endl; } return 0; }
Since the given string in str
starts with the specified prefix string, the if-condition evaluates to true, and the if-block runs.
Output
The string starts with the prefix.
Now, let us change the prefix value, and run the program again.
main.cpp
#include <iostream> #include <string> using namespace std; int main() { string str = "Hello World"; string prefix = "Apple"; if (str.substr(0, prefix.length()) == prefix) { cout << "The string starts with the prefix." << endl; } else { cout << "The string does not start with the prefix." << endl; } return 0; }
Since the given string in str
does not start with the specified prefix string, the if-condition evaluates to false, and the else-block runs.
Output
The string does not start with the prefix.
2. Checking if the string starts with a specific prefix using string compare() method in C++
In the following program, we take a string in str
and a prefix string value in prefix
. We have to check if the string str
starts with the prefix string prefix
using string compare() method.
Steps
- Given an input string in
str
, and a prefix string inprefix
. - Use the string
compare()
method to compare the substring ofstr
starting from index 0 and having a length equal to the length ofprefix
with theprefix
string. - If the value returned by
compare()
method is 0, then the stringstr
starts with the specified prefix value. Else the stringstr
does not start with the specified prefix value.
Program
main.cpp
#include <iostream> #include <string> using namespace std; int main() { string str = "Hello World"; string prefix = "Hello"; if (str.compare(0, prefix.length(), prefix) == 0) { cout << "The string starts with the prefix." << endl; } else { cout << "The string does not start with the prefix." << endl; } return 0; }
Output
The string starts with the prefix.
Conclusion
In this C++ Tutorial, we learned how to check if the string starts with the specified prefix value using string compare() method, or string substr() method and string equality check, with examples.