Go For Loop
Go For Loop is used to iterate a set of statements based on a condition or over a range of elements.
In this tutorial, we will learn the syntax of For Loop in Go programming, and understand how to use for loop in different scenarios.
You can use for loop in three forms. They are
1. For Loop with Condition
The syntax of for loop in Go, with just condition alone is
for condition {
statement(s)
}
An example for For Loop with condition is shown below.
example.go
package main
import "fmt"
func main() {
var i int = 0
for i<5 {
fmt.Println(i)
i = i+1
}
}
We have to initialize the control variable, i
before the for loop and update it inside the for loop.
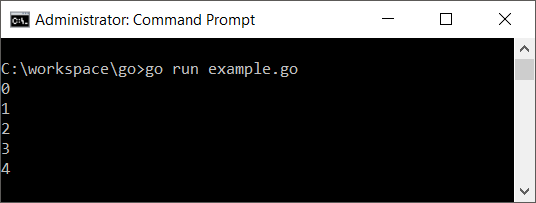
2. For Loop with Initialization, Condition and Update
The syntax of Go For Loop statement, with initialization, condition and update sections is
for init; condition; update {
//code
}
An example for For Loop with initialization, condition and update is shown below.
example.go
package main
import "fmt"
func main() {
for i:=0; i<5; i++ {
fmt.Println(i)
}
}
The initialization and update of the control variable i
happens in the for statement iteslf. This form of for loop is recommended as you would not forget the initialization and update of control variable, which otherwise could result in an infinite loop sometimes.
3. For Loop over a Range
The syntax of For loop to iterate over a Range is
for x in range {
statement(s)
}
An example for For Loop over a range is shown below.
example.go
package main
import "fmt"
func main() {
a := [6]int{52, 2, 13, 35, 9, 8}
for i,x:= range a {
fmt.Printf("a[%d] = %d\n", i,x)
}
}
We can access both the index and the element when range is iterated using a for loop. In this example, we get index to i
and the element to x
during each iteration.
Conclusion
In this Go Tutorial, we learned about For Loop in Go, and its different forms for different types of data and scenarios.