Go Functions
Functions are fundamental building blocks in Go, enabling modularity, reusability, and clean organization of code.
In this tutorial, we will discuss the syntax, key components, and various types of functions in Go, with examples and detailed explanations.
Basic Function Syntax
The basic syntax for defining a function in Go is as follows:
</>
Copy
func functionName(parameters) returnType {
// Function body
}
Key components of a function:
func
: Keyword to define a function.- functionName: The name of the function, which is used to call it.
- parameters: A list of input variables with their types (optional).
- returnType: The type of value the function returns (optional).
- Function body: The block of code that executes when the function is called.
Types of Functions
Go supports various types of functions. Let’s explore each type with examples:
1 Function Without Parameters and Return Value
A simple function that does not take any parameters or return a value:
</>
Copy
package main
import "fmt"
// Function without parameters and return value
func greet() {
fmt.Println("Hello, World!")
}
func main() {
greet() // Call the function
}
Explanation
- Define Function: The function
greet
is defined without parameters or return value. - Print Message: The function body contains a statement to print a message.
- Call Function: The function is called in the
main
function to execute its body.
Output
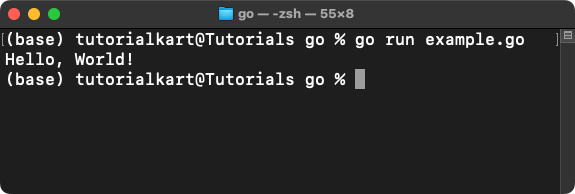
2 Function With Parameters
Functions can take input parameters:
</>
Copy
package main
import "fmt"
// Function with parameters
func add(a int, b int) {
fmt.Println("Sum:", a+b)
}
func main() {
add(10, 20) // Call the function with arguments
}
Explanation
- Define Function: The function
add
takes two parametersa
andb
, both of typeint
. - Perform Addition: The function calculates the sum of
a
andb
. - Call Function: The function is called in
main
with arguments10
and20
.
Output
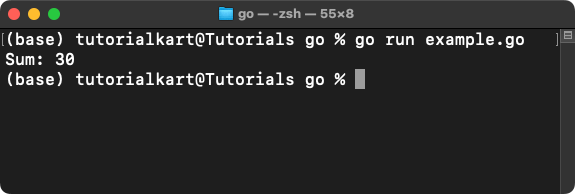
3 Function With Return Value
A function can return a value to the caller:
</>
Copy
package main
import "fmt"
// Function with return value
func multiply(a int, b int) int {
return a * b
}
func main() {
result := multiply(5, 4) // Call the function and store the result
fmt.Println("Product:", result)
}
Explanation
- Define Function: The function
multiply
takes two parameters and returns an integer. - Return Product: The product of the two parameters is returned using the
return
keyword. - Call Function: The function is called in
main
, and the result is stored inresult
.
Output
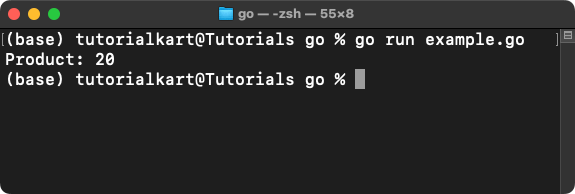
4 Function With Multiple Return Values
Go supports functions that return multiple values:
</>
Copy
package main
import "fmt"
// Function with multiple return values
func divide(a int, b int) (int, int) {
return a / b, a % b // Return quotient and remainder
}
func main() {
quotient, remainder := divide(10, 3) // Call the function and capture results
fmt.Println("Quotient:", quotient)
fmt.Println("Remainder:", remainder)
}
Explanation
- Define Function: The function
divide
takes two parameters and returns two integers. - Return Values: The quotient and remainder are calculated and returned.
- Call Function: The function is called in
main
, and the results are stored inquotient
andremainder
.
Output
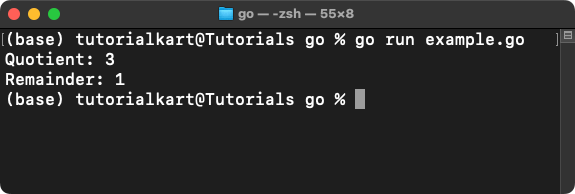
5 Variadic Function
A variadic function can accept a variable number of arguments:
</>
Copy
package main
import "fmt"
// Variadic function
func sum(nums ...int) int {
total := 0
for _, num := range nums {
total += num
}
return total
}
func main() {
fmt.Println("Sum:", sum(1, 2, 3, 4, 5)) // Call with multiple arguments
}
Explanation
- Define Variadic Function: The function
sum
accepts a variable number of integers usingnums ...int
. - Iterate Over Arguments: The function iterates over all arguments using a
for
loop. - Return Sum: The total sum of the arguments is returned.
Output
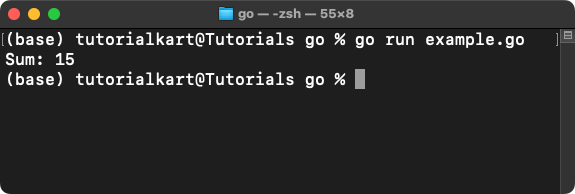
Key Notes
- Functions in Go must start with the
func
keyword. - Functions can have parameters, return values, both, or neither.
- Function names starting with an uppercase letter are exported and can be used outside their package.
- Variadic functions allow you to pass multiple arguments of the same type.