In this Java tutorial, you will learn what inheritance is when it comes to Object Oriented Programming, and how Inheritance is implemented in Java with classes, with examples.
Inheritance
Inheritance is an Object Oriented Concept in Java. It allows an object of a class to own the variables and methods of another class. In Java, Inheritance is realized using the keyword extends.
In a parent-child analogy, child inherits parents variables(money, house, etc.,) and methods(behaviors from genetics). The same way, an object of a child class, which extends a Parent class, can access the variables and methods of Parent class as of its own.
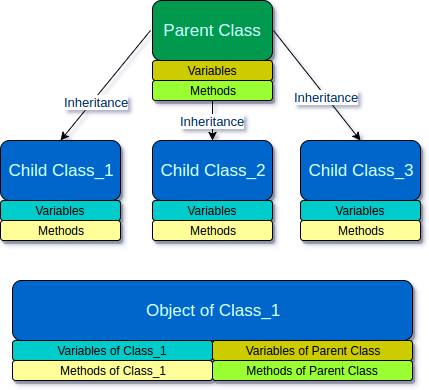
More about inheritance
The following are the most important list of points to remember about the Java Inheritance concept.
- Inheritance is an Object Oriented Programming(OOP) concept.
- A Child class can inherit only one Parent class. (A child can have only one parent)
- Multiple (sub) classes can inherit a same (super) class. (A parent can have multiple children)
- Child class may use the methods and variables of the Parent class.
- A child class can inherit all methods of parent class except those which are priavte.
When a Child class inherits a Parent class, compiler does not copy the methods of Parent class to Child class. But, the compiler makes a reference to the methods in the instance of Parent class.
Example Program
Usually in Java, Parent class is also called as Super class, and Child class is also called as Sub Class.
In this example project, we shall create a Super Class, MobilePhone.java
and a Sub Class SmartPhone.java
.
Following is the parent class or super class.
MobilePhone.java
package com.tutorialkart.java;
/**
* @author tutorialkart
*
*/
public class MobilePhone {
public int price;
public void makeCall(){
System.out.println("Calling...");
}
public void getCharge(){
System.out.println("Charging...");
}
// private method is not visible to other classes
private void check(){
System.out.println("This is private to MobilePhone");
}
}
Following is the child class or subclass.
SmartPhone.java
package com.tutorialkart.java;
/** This class inherits MobilePhone
* @author tutorialkart
*/
public class SmartPhone extends MobilePhone {
public String name;
public static void main(String[] args) {
SmartPhone sonyXZ = new SmartPhone();
// variable of SmartPhone (Sub Class)
sonyXZ.name = "Sony XZ";
// variable of MobilePhone (Super Class)
sonyXZ.price = 40500;
// method of SmartPhone (SubClass)
sonyXZ.playVideo();
// methods of MobilePhone (Super Class)
sonyXZ.makeCall();
sonyXZ.getCharge();;
}
public void playVideo(){
System.out.println("Playing video..");
}
}
Run SmartPhone.java program, and the output to console should be as shown in the following.
Output
Playing video..
Calling...
Charging...
Conclusion
In this Java Tutorial, we learned what Inheritance mean in Java and how to realize it using extends keyword.