Java – Download File from URL/Website
To download a file from internet using URL, you can use FileUtils.copyURLToFile() method of org.apache.commons.io package.
You can download any type of File using this method. You can download a HTML page, PNG image, JPEG image, JavaScript js file, Documents hosted on cloud, etc.
In this tutorial, we will learn the sequence of steps to save the file from URL.
Following is the step by step process to download file from URL in Java.
- Prepare an URL object with the URI passed as argument to URL() class.
- Prepare a File object with the path of the destination at which the file has to be saved.
- Call the method FileUtils.copyURLToFile() with the URL and File objects, prepared in the above steps, passed as arguments.
Examples
1. Download File from URL
In this example, we will download the HTML file that served for the URL “https://www.tutorialkart.com/”.
DownloadFromURL.java
import java.io.File; import java.io.IOException; import java.net.MalformedURLException; import java.net.URL; import org.apache.commons.io.FileUtils; /** * Java Example Program to download file from website */ public class DownloadFromURL { public static void main(String[] args) { try { URL url = new URL("https://www.tutorialkart.com/"); File destination_file = new File("files/tutorialkart.html"); FileUtils.copyURLToFile(url, destination_file); } catch (MalformedURLException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } } }
new URL()
throws MalformedURLException. We have to handle the exception using a catch block.
FileUtils.copyURLToFile()
throws IOException. We have to handle the exception using a catch block.
We have enclosed our code block in a try catch block with both the above said exceptions handled.
When you run the program, if everything is good, meaning, the URL is formed correctly, and the FileUtils has created and saved the content to a file, the file is created at the specified destination.
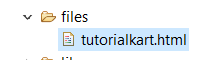
2. Download Image File from URL
Like an HTML page in the previous example, you can also download any type of file.
In this example, we will download an image from URL and save it in our local file system.
DownloadFromURL.java
import java.io.File; import java.io.IOException; import java.net.MalformedURLException; import java.net.URL; import org.apache.commons.io.FileUtils; /** * Java Example Program to download an image from URL */ public class DownloadFromURL { public static void main(String[] args) { try { URL url = new URL("https://www.tutorialkart.com/img/tutorials.png"); File destination_file = new File("files/tutorials.png"); FileUtils.copyURLToFile(url, destination_file); } catch (MalformedURLException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } } }
Run the program, and you shall see an image file tutorials.png
downloaded to the specified location using File object.
Conclusion
In this Java Tutorial, we learned how to download a File from URL in Java using FileUtils class.