Java Program – Check if Number is Even or Odd
Solution: To check if a natural number is even or odd, we have to divide the number by 2 and find the reminder. If the reminder is zero, then the number is even, else it is false.
When you divide a natural number by 2, the reminder has only two possible values, which are either zero or one. So, you can either check for the condition that reminder is zero, or reminder is one. To find the reminder, we shall use the modulus operator in Java.
In this tutorial, we shall write Java programs using if-else conditional statement, to decide if a number is even or odd.
Example 1 – Check if Number is Even or Odd
In the following Java program, we shall read a number from console entered by user in standard input. Then we shall check if this number is even or odd using Java If-Else statement.
If a
is the variable in which we are reading an integer, then a%2
finds the reminder when a
is divided by 2
. To check if this reminder is equal to 0
, we shall use equal to operator ==
. Thus our condition becomes a%2==0
to check if a
is even. If this condition returns true, then a
is even
, else a
is odd
.
Example.java
import java.util.Scanner; /** * Java Program - Check if Number is Even or Odd */ public class Example { public static void main(String[] args) { //create a scanner to read bytes from console entered by user via keyboard Scanner scanner = new Scanner(System.in); System.out.print("Enter a number : "); //read integer from user int a = scanner.nextInt(); //check if the number is even or odd if(a%2==0) { System.out.println("The number is even."); } else { System.out.println("The number is odd."); } //close the scanner scanner.close(); } }
Run the above program, and enter a number in the console. You shall get an output similar to the following, based on the number you entered.
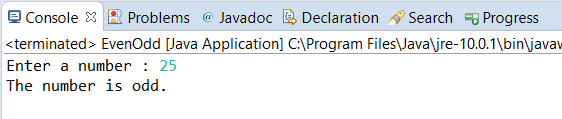
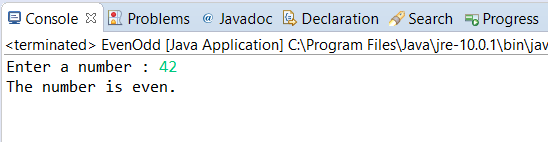
Example 2 – Check if Number is Odd or Even
Let us now check if the number is odd in the condition. And then in the else block, we conclude that the number is even.
Example.java
import java.util.Scanner; /** * Java Program - Check if Number is Even or Odd */ public class Example { public static void main(String[] args) { //create a scanner to read bytes from console entered by user via keyboard Scanner scanner = new Scanner(System.in); System.out.print("Enter a number : "); //read integer from user int a = scanner.nextInt(); //check if the number is even or odd if(a%2==1) { System.out.println("The number is odd."); } else { System.out.println("The number is even."); } //close the scanner scanner.close(); } }
Similar to the previous example, when you run the program, the console prompts user to enter a number. Enter a number and the program shall continue with the execution to decide if given number is odd or even.
Output
Enter a number : 89 The number is odd.
Conclusion
In this Java Tutorial, we have learned how to check if a number is even or odd with the help of example Java programs.