In this Java tutorial, you will learn how to read an integer from console input entered by user using Scanner.nextInt() method, with examples.
Read Integer from Console in Java
In Java, you can read an integer from Console using nextInt() on Scanner.
In this tutorial, we will learn how to read an integer from user input via console.
First of all, to read anything from the console, you have to create a Scanner with System standard input of the computer.
Then use nextInt() method on the scanner object to read an integer from the console.
Examples
1. Read integer from console and print it
In the following Java program, we read an integer from console input and print it back to the console.
Example.java
import java.util.Scanner;
public class Example {
public static void main(String[] args) {
// Create a scanner to read bytes from standard input
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a number : ");
// Read integer from console entered via standard input
int num = scanner.nextInt();
System.out.println("You entered the number : "+ num);
// Close scanner
scanner.close();
}
}
Run this program. When it is run, it asks user to enter a number.
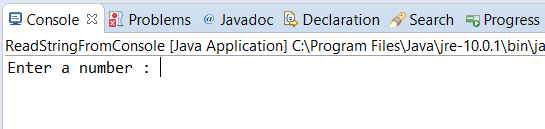
If the user enters a number, scanner.nextInt() reads the input to an integer.
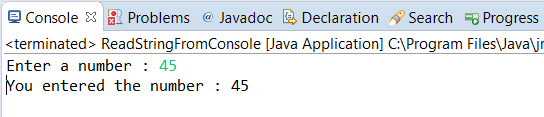
If you do not provide an integer in the console input, you will get InputMismatchException as shown below.
Enter a number : 52ds
Exception in thread "main" java.util.InputMismatchException
at java.base/java.util.Scanner.throwFor(Unknown Source)
at java.base/java.util.Scanner.next(Unknown Source)
at java.base/java.util.Scanner.nextInt(Unknown Source)
at java.base/java.util.Scanner.nextInt(Unknown Source)
at JavaTutorial.main(JavaTutorial.java:10)
It is because, we are trying to read an integer, but actually got a string that is not a valid number.
Conclusion
In this Java Tutorial, we learned how to read an integer from console.