Java – Create a File
To create a file in Java, we can use Files.write() function. We need to pass the path of the file, and the content as arguments to write() function.
In this tutorial, we will learn how to create a file and write some text into it.
1. Create a file using Files.write()
In this example, we will use Files.write() function to create a file at the given path, and write a string to it.
Note: Make sure the the directory we specified in the path
is present. If the directory is not present, then write() function throws java.nio.file.NoSuchFileException
.
Main.java
</>
Copy
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class Main {
public static void main(String[] args) {
Path filePath = Paths.get("data/sample.txt");
String content = "Hello World";
try {
Files.write(filePath, content.getBytes());
System.out.println("File created successfully.");
} catch (IOException e) {
e.printStackTrace();
System.out.println("Issue in creating file.");
}
}
}
Run the program from command prompt or in your favourite IDE like Eclipse.
Output
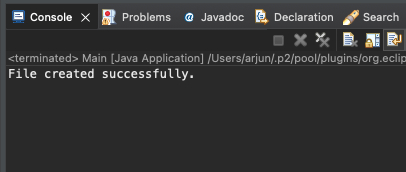
Created file
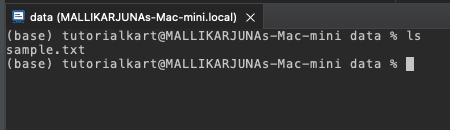
Conclusion
In this Java Tutorial, we learned how to create a file in Java, using Files.write() function.