In this tutorial, you will learn about classes and objects in Java. About the properties and methods of a class, how to create objects of a class, etc.
Classes
In Java, Class is what describes the properties and routines, an object could possess. In other words, “Class is a blueprint”.
Properties are variables in a class, that define the state of an object during its life time.
Routines are methods in a class, which define the behavior or tasks that an object can do.
Class Example
We see lot of objects in the real world. For example, Consider XY type of cars. There are lots of cars belonging to XY type which are analogous to objects. Since they have a blueprint, these XY cars are identical to each other. This blueprint, to which the manufacturers look up to, could be thought of a class in Java for understanding.
- Properties could be current level of fuel, speed, head lights on/off, etc.,
- Routines could be moving, breaking, etc.,
Following is an example of a class in Java.
CarClass.java
package com.tutorialkart.java; public class CarClass { public final String carManufacturer = "XY"; public int milesRun; public float velocity; public void run(){ milesRun++; velocity = 20.0f; }; public void applyBreak(){ velocity = 0.0f; } }
Following is the detailed view of components in the class.
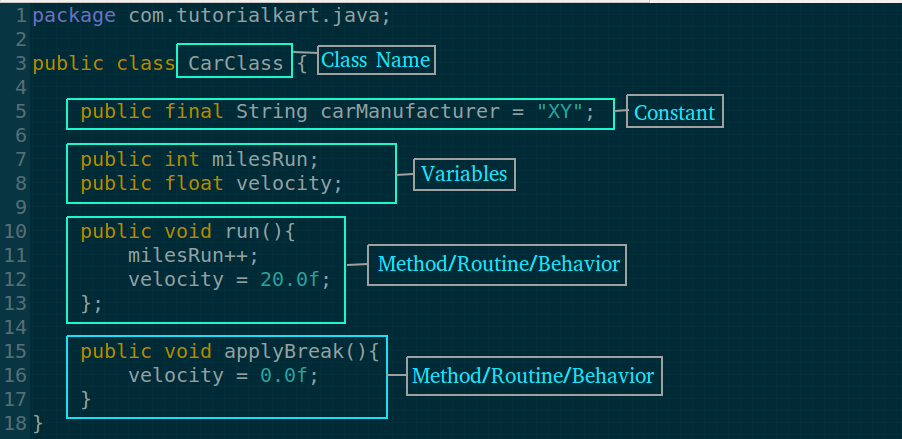
Objects
Create objects of a class
The keyword, new, is used to create an object. Let us see how to create an object of class type CarClass
that is defined above Java class example.
CarClass car1 = new CarClass();
Following picture depicts the components for declaring and creating a new object of class CarClass
.
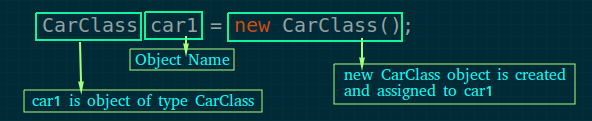
Access properties and methods of an object
You can access properties and methods of a class using dot operator.
In the following example program, an object of type CarClass
is created. Its variable milesRun
is assigned a value using dot (.) operator. Methods of the object, run()
and applyBreak()
are called using dot(.) operator.
Traffic.java
package com.tutorialkart.java; public class Traffic { public static void main(String[] args) { CarClass car1 = new CarClass(); car1.milesRun = 0; car1.run(); System.out.println("car1 is running.."); System.out.println("Miles run by car1 : "+car1.milesRun); System.out.println("Velocity of car1 : "+car1.velocity); car1.applyBreak(); System.out.println("Applying break to car1.."); System.out.println("Velocity of car1 after applying : "+car1.velocity); } }
Note : CarClass.java and Traffic.java are in same package com.tutorialkart.java
, which is the reason for not importing the CarClass.java in Traffic.java.
When the above program Traffic.java
is run, the result is as shown in the following.
car1 is running.. Miles run by car1 : 1 Velocity of car1 : 20.0 Applying break to car1.. Velocity of car1 after applying : 0.0
This is how we define classes in Java and create object for a Class.
Conclusion
In this Java Tutorial, we learned how to define a Java Class; create objects for a Class; access properties and call methods on objects using dot operator; etc.
In our next tutorial, we shall learn about Inheritance in Java.