Java Program – Add Two Integers
Adding two integers is an arithmetic operation. So, we shall use Arithmetic Addition Operator +
to perform addition of two integers in Java.
In this tutorial, we shall write Java Programs to add two integers, covering different realtime situations.
Coming to first example, we shall take two hard coded integers and find their sum using arithmetic addition operator.
In the second example, we shall read two integers from console which shall be entered by user, and find their sum.
Example 1 – Add Two Integers
In this example, we shall take two integers and find their sum using arithmetic operator.
AddTwoIntegers.java
/**
* Java Program - Add Two Integers
*/
public class AddTwoIntegers {
public static void main(String[] args) {
//two numbers
int num1 = 10;
int num2 = 25;
int sum;
//add two numbers
sum = num1 + num2;
//print the sum
System.out.println(sum);
}
}
Explanation
int num1 = 10;
declares num1
as integer and initializes num1
with 10
.
int num2 = 25;
declares num2
as integer and initializes num2
with 25
.
int sum;
declares sum as integer. We shall use this variable to store the sum of the the two numbers num1
and num2
.
num1 + num2
: We are using arithmetic addition operator with num1
and num2
as operands. The addition operator adds num1
and num2
, and returns the resulting value. We are storing the returned value in the variable sum
. Finally, we are getting the result of addition of the two numbers in the variable sum
.
System.out.println(sum);
prints the value stored in variable sum
to console.
Run the above Java program, and you shall get the following output.
Output
35
Example 2 – Read Integers from Console and Find their Sum
In this example, we shall take two integers entered by the user in console, and find their sum using arithmetic operator.
AddTwoIntegers.java
import java.util.Scanner;
/**
* Java Program - Add Two Integers
*/
public class AddTwoIntegers {
public static void main(String[] args) {
//initialize scanner that scans input entered by user in console
Scanner myInput = new Scanner( System.in );
//read two numbers from console
System.out.print("Enter first number : ");
int num1 = myInput.nextInt();
System.out.print("Enter second number : ");
int num2 = myInput.nextInt();
int sum;
//add two numbers
sum = num1 + num2;
//print the sum
System.out.println(sum);
//close the scanner
myInput.close();
}
}
Explanation
Scanner myInput = new Scanner( System.in ); initializes scanner for system standard input (keyboard). So, when user enters numbers in the console using keyboard, this Scanner allows us to read those numbers.
System.out.print("Enter first number : ");
prints the string to the console, so that user is informed what to do, that is entering first number using keyboard.
int num1 = myInput.nextInt();
declares num1
as integer and initializes with value entered by user in the console using keyboard. User has to enter the number and press enter button to mark end of the integer.
System.out.print("Enter second number : ");
prints the string to the console, so that user is informed what to do, that is entering second number using keyboard.
int num2 = myInput.nextInt();
declares num2
as integer and initializes with value entered by user in the console using keyboard. User has to enter the number and press enter button to mark end of the integer.
int sum;
declares sum as integer. We shall use this variable to store the sum of the the two numbers num1
and num2
.
num1 + num2
: We are using arithmetic addition operator with num1
and num2
as operands. The addition operator adds num1
and num2
, and returns the resulting value. We are storing the returned value in the variable sum
. Finally, we are getting the result of addition of the two numbers in the variable sum
.
System.out.println(sum);
prints the value stored in variable sum
to console.
myInput.close();
Finally, we shall close the Scanner.
Run the above Java program, and you shall get the following output.
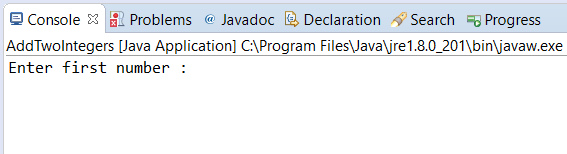
Enter a number using keyboard and click enter (in Windows) or return (in Mac).
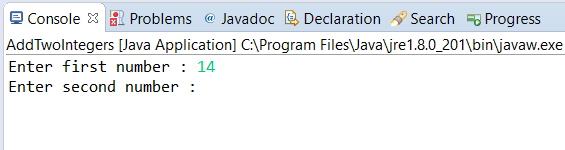
Enter a number using keyboard and click enter (in Windows) or return (in Mac).
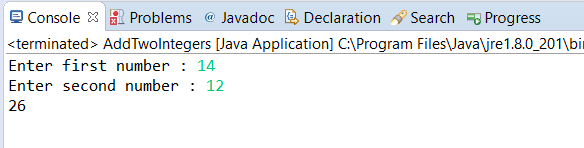
Conclusion
Concluding this Java Tutorial, we have written Java Program to add two integers with detailed explanation for each example.