In this tutorial, you will learn how to check if a file exists in Java, using exists() method of java.io.File class.
Java – Check if file exists
To check if a file exists in Java, create a File object using the given file path, and call exists() method on the file object. If the file is present, then exists() method returns true, or else, it returns false.
If file
is the File object created from the given file path, then use the following expression to check if the file exists.
file.exists()
As already mentioned, if the file exists at given path, then exists() method returns true. Or if the file does not exist at given file path, then exists() method returns false.
1. Check if the file at given path exists in Java
In the following program, we take a file path "/Users/tutorialkart/data.txt"
such that the file exists. We use File exists() method to programmatically check if the file is present at given path or not.
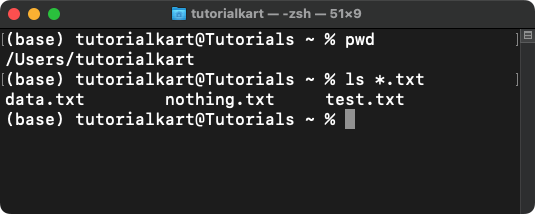
Java Program
import java.io.File; public class Main { public static void main(String[] args) { String filePath = "/Users/tutorialkart/data.txt"; File file = new File(filePath); if (file.exists()) { System.out.println("FILE EXISTS."); } else { System.out.println("FILE DOES NOT EXIST."); } } }
Output
FILE EXISTS.
2. Check if the file “dummy.txt” exists at given path in Java
In the following program, we take a file path "/Users/tutorialkart/dummy.txt"
such that the file does not exist. We use File exists() method to programmatically check if the file is present at given path or not.
Java Program
import java.io.File; public class Main { public static void main(String[] args) { String filePath = "/Users/tutorialkart/dummy.txt"; File file = new File(filePath); if (file.exists()) { System.out.println("FILE EXISTS."); } else { System.out.println("FILE DOES NOT EXIST."); } } }
Output
FILE DOES NOT EXIST.
Conclusion
In this Java File Operations tutorial, we learned how to check if a file at given path exists or not using File exists() method.