Java System.setErr() – Examples
In this tutorial, we will learn about the Java System.setErr() function, and learn how to use this function to set standard error output stream for the System, with the help of examples.
setErr(PrintStream err)
System.setErr() Reassigns the “standard” error output stream.
Syntax
The syntax of setErr() function is
setErr(PrintStream err)
where
Parameter | Description |
---|---|
err | The new standard error output stream for the System. |
Returns
The function returns void.
Example 1 – setErr()
In this example, we will set a FileOutputStream as the System standard output using setErr(). Any values that are printed to standard error output, will be written to the file errors.txt
specified by FileOutputStream object f
.
If the specified file is not present, new file would be created.
Java Program
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.PrintStream;
public class Example {
public static void main(String[] args) throws FileNotFoundException {
FileOutputStream f = new FileOutputStream("errors.txt");
System.setErr(new PrintStream(f));
System.err.println("Hello World!");
}
}
Output – errors.txt
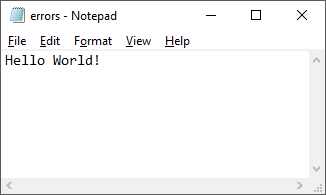
Example 2 – setErr() – Null PrintStream
In this example, we will set a null value, for PrintStream object, and set this as the System standard error output stream using setError(). Since the argument to setErr() is null, the method throws java.lang.NullPointerException.
Java Program
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.PrintStream;
public class Example {
public static void main(String[] args) throws FileNotFoundException {
System.setErr(null);
System.err.println("Hello World!");
}
}
Output
Exception: java.lang.NullPointerException thrown from the UncaughtExceptionHandler in thread "main"
Conclusion
In this Java Tutorial, we have learnt the syntax of Java System.setErr() function, and also learnt how to use this function with the help of examples.