Press Browser’s Forward Button
To press the browser’s forward button using Selenium for Java, call navigate().forward()
on the WebDriver object.
The following is a simple code snippet to simulate the pressing of browser’s forward button.
driver.navigate().forward();
where
ADVERTISEMENT
driver
is a WebDriver object.driver.navigate()
returns the Navigation object that allows functionalities like back, forward, refresh, etc.driver.navigate().forward()
simulates the clicking of the browser’s forward button, and returns void.
Example
In the following program, we write Selenium Java code to visit Tutorialkart Home Page, click on Java Tutorial link with href=”/java/”, press the browser’s back button using driver.navigate().back()
, and then simulating the pressing of browser’s forward button using driver.navigate().forward()
.
Java Program
import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; public class MyAppTest { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver"); WebDriver driver = new ChromeDriver(); try { driver.get("https://www.tutorialkart.com/"); //click on Java link driver.findElement(By.xpath("//a[@href=\"/java/\"]")).click(); //press on Browser's back button driver.navigate().back(); //press on Browser's forward button driver.navigate().forward(); } finally { driver.quit(); } } }
Screenshots
1. Load Tutorialkart Home page.
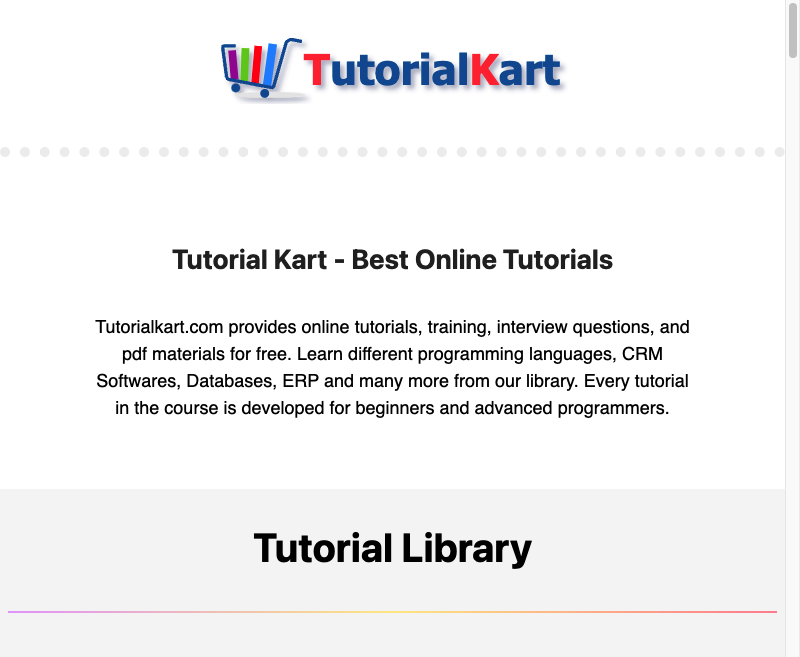
2. Click on Java link.
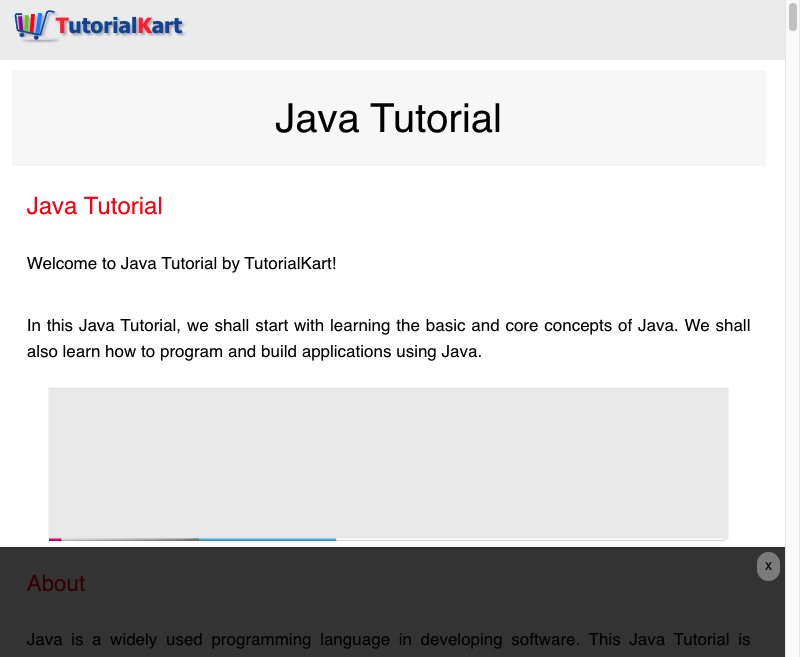
3. Press Browser’s back button.
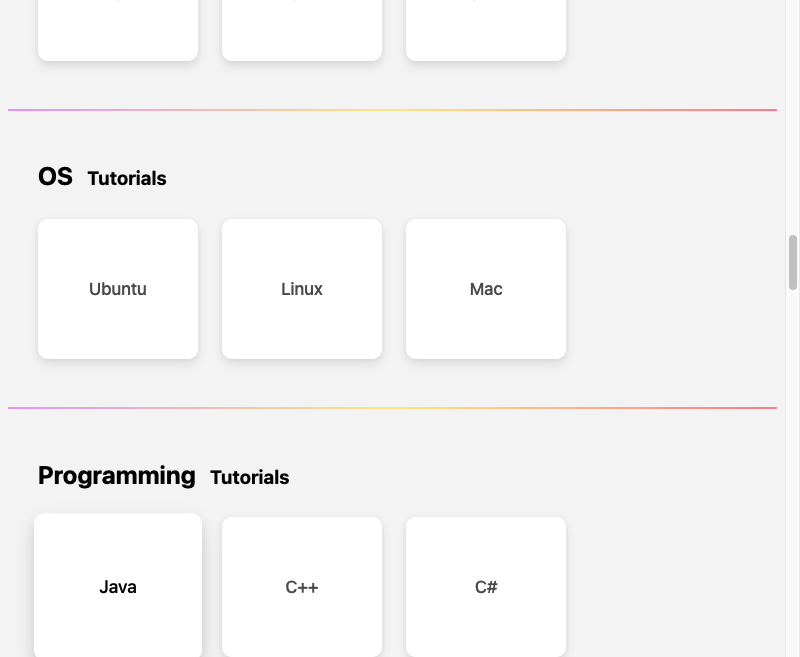
4. Press Browser’s forward button.
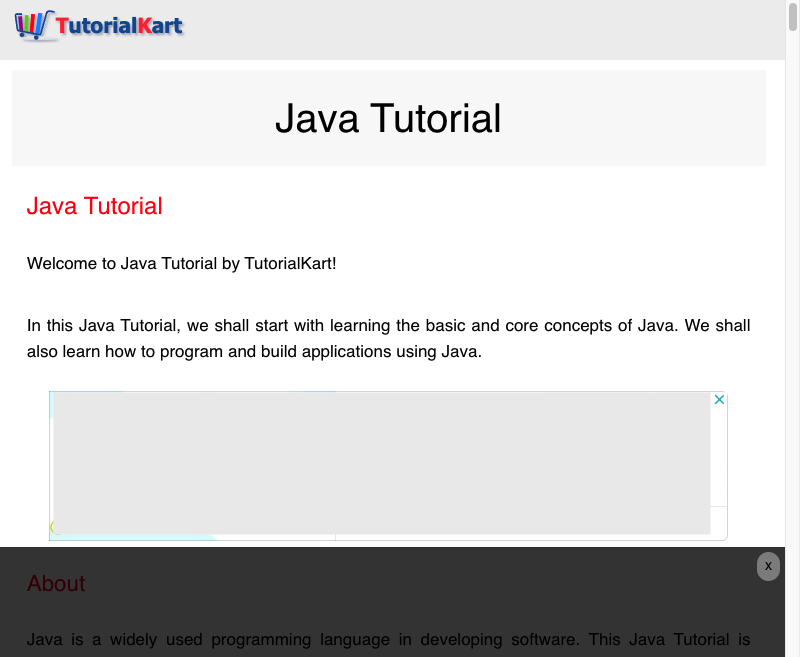