Take Screenshot of Current Browser Window
To take the screenshot of the current browser window programmatically using Selenium in Java, use driver as TakesScreenshot
instance and call getScreenshotAs()
method on this instance. Specify the output type as FILE in argument to getScreenshotAs()
method.
getScreenshotAs(OutputType.FILE)
method returns the File object. We have to save it to the local storage as a PNG or JPG file.
The following is a simple code snippet to initialize a web driver and maximize the window.
File screenshot = ((TakesScreenshot)driver).getScreenshotAs(OutputType.FILE); FileUtils.copyFile(screenshot, new File("images/some-file-name.png"));
Note: We have used FileUtils.copyFile()
method of org.apache.commons.io
package to save the file to local storage.
Example
In the following program, we write Java code to initialize a web driver, get a URL, and take the screenshot of the current browser window.
Java Program
import java.io.File; import java.io.IOException; import org.apache.commons.io.FileUtils; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; public class MyAppTest { public static void main(String[] args) { //initialize web driver System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver"); WebDriver driver = new ChromeDriver(); try { //get the URL driver.get("https://www.tutorialkart.com/"); //take screenshot File screenshot = ((TakesScreenshot)driver).getScreenshotAs(OutputType.FILE); FileUtils.copyFile(screenshot, new File("images/my-screenshot.png")); } catch (IOException e) { e.printStackTrace(); } finally { driver.quit(); } } }
Screenshot – images/my-screenshot.png
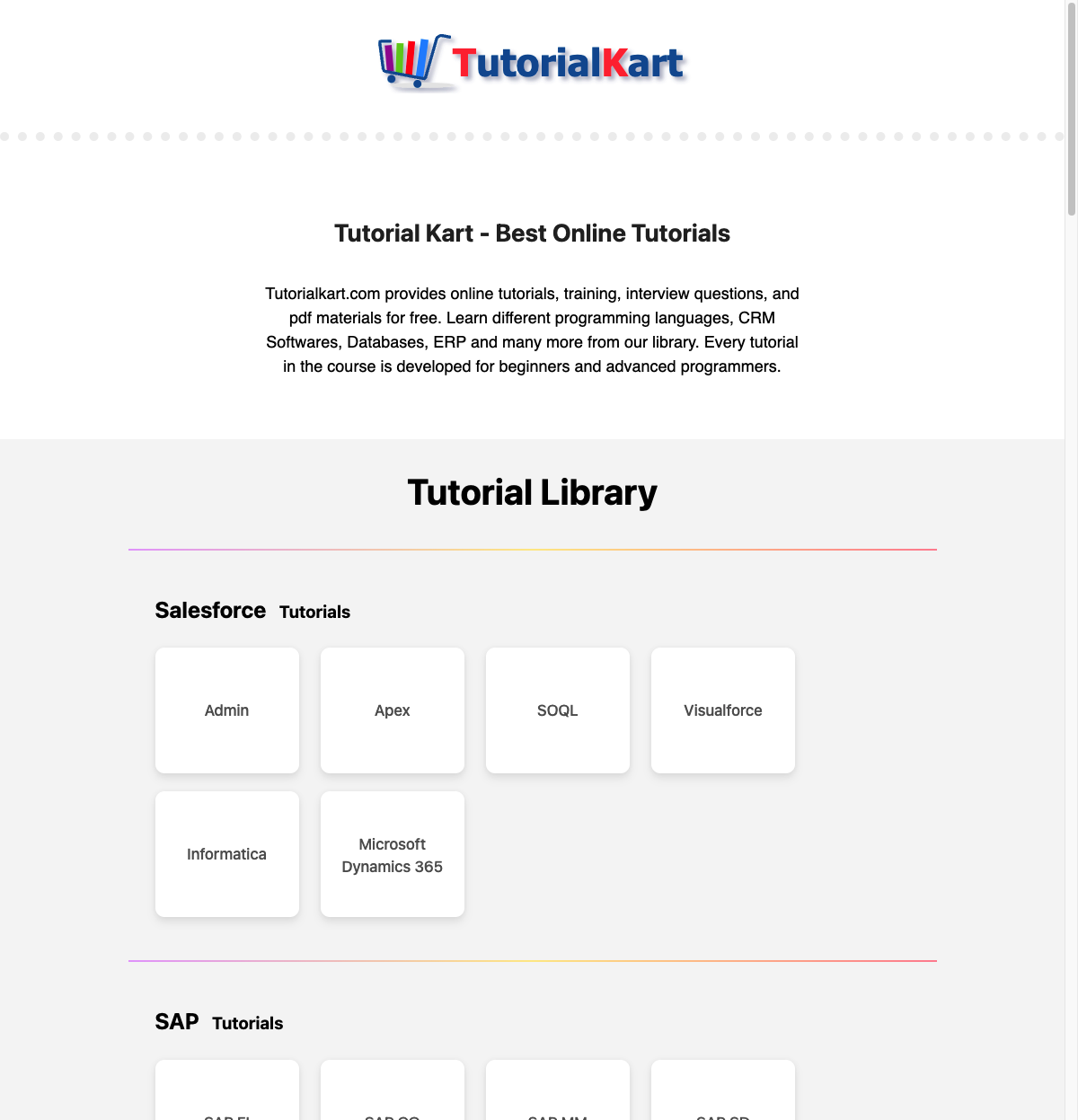