In this tutorial, you will learn how to get the size of a file in Java, using size() method of java.nio.file.Files class.
Java – Get file size
To get the size of a file given by a path in Java, prepare Path object for the given file, call size() method of the java.nio.file.Files class and pass the file path object as argument to the method. The size() method returns a number (of long type) representing the size of the given file.
If filePath
is the given file path, then use the following expression to get the size of file.
Files.size(filePath)
1. Get the size of file “data.txt” in Java
In the following program, we take a file path "/Users/tutorialkart/data.txt"
, get the size of this file, and print the size to standard output.
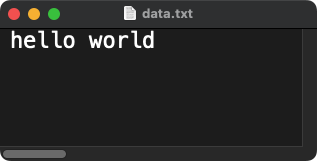
In the program,
- Create a Path object filePath from the given file path.
- Pass the file path object to size() method of the java.nio.file.Files class.
- The size() method returns the size of the file in bytes, as long value. Store it in variable fileSize.
- You may print the file size to output using println() method.
- If there is IOException while handling the file, you may address it using a Java Try Catch statement.
Java Program
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
try {
// Prepare file path
Path filePath = Paths.get("/Users/tutorialkart/data.txt");
// Get size of the file
long fileSize = Files.size(filePath);
System.out.println("File size : " + fileSize);
} catch (IOException e) {
System.out.println("An error occurred: " + e.getMessage());
}
}
}
Output
File size : 12
Conclusion
In this Java File Operations tutorial, we learned how to get the file size using java.nio.file.Files size() method, with examples.