In this tutorial, you will learn how to read a file as string from resources in Java, using ClassLoader, InputStream, and Scanner classes.
Java – Read file as string from resources
To read a file as a string from resources in Java,
- Given a path to the file in resources.
- Get the file from resources as an InputStream using ClassLoader.getResourceAsStream() method.
- Use Scanner class and read the contents of the InputStream to a string.
Make sure there is resources folder added to the classpath. Refer Add resources to Java Project in Eclipse tutorial, to know the step by step process of adding a resources folder to your Java Project in Eclipse IDE.
1. Read the file “data.txt” as string from resources in Java
In the following program, we take a file path "data.txt"
which is present in the resources folder.
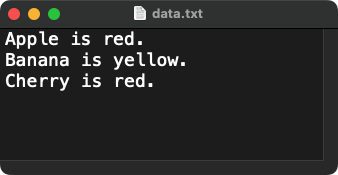
In the program,
- Given a file path filePath as String.
String filePath = "data.txt";
- Use ClassLoader and get the resource (file identified by given filePath) as InputStream.
InputStream inputStream = Main.class.getClassLoader().getResourceAsStream(filePath);
Please note that the class Main
is the name of our Java class, in which we are writing our program. If you are using another name, then please replace Main
with your class name.
- Read the content from the inputStream to Scanner in one go using useDelimiter() method.
Scanner scanner = new Scanner(inputStream).useDelimiter("\\A");
- Read content from Scanner to a string using next() method of Scanner instance. We shall use a Java Ternary Operator to check if the Scanner has content, and then assign the content to a string. If Scanner does not have any content, then we shall consider an empty string.
String fileContent = scanner.hasNext() ? scanner.next() : "";
- You may print the fileContent to output.
System.out.println(fileContent);
- Close the inputStream and scanner.
inputStream.close();
scanner.close();
The following is the complete Java program, to read the file as InputStream, and then print the content of the InputStream.
Java Program
import java.io.IOException;
import java.io.InputStream;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
String filePath = "data.txt"; // Path relative to the resources folder
try {
// Open an InputStream for the resource using the ClassLoader
InputStream inputStream = Main.class.getClassLoader().getResourceAsStream(filePath);
if (inputStream != null) {
// Use a Scanner to read the contents of the InputStream
Scanner scanner = new Scanner(inputStream).useDelimiter("\\A");
String fileContent = scanner.hasNext() ? scanner.next() : "";
// Print the file content
System.out.println(fileContent);
// Close the resources
inputStream.close();
scanner.close();
} else {
System.out.println("Resource not found: " + filePath);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output
Apple is red.
Banana is yellow.
Cherry is red.
Conclusion
In this Java File Operations tutorial, we learned how to read the file as a string from resources using ClassLoader, InputStream, and Scanner classes, with examples.