Express.js Router
Express.js Router is kind of nesting a mini server inside a server.
Create an Express.js Router
In the following example, we will create an API using router. The API is created separately to demonstrate modularity.
router1.js
var express = require('express')
var router1 = express.Router()
// middleware that is specific to this router
router1.use(function timeLog (req, res, next) {
console.log('Requested URI Path : ', req.url)
next()
})
// define the home page route
router1.get('/', function (req, res) {
res.send('Birds home page')
})
// define the about route
router1.get('/about', function (req, res) {
res.send('About birds')
})
module.exports = router1
We created a router using express.Router()
and then created some routing paths
app.js
var express = require('express')
var app = express()
var router1 = require('./router1')
app.use('/api/', router1)
// start the server
var server = app.listen(8000, function(){
console.log('Listening on port 8000...')
})
When we used app.use('/api/', router1)
all the requests to the server with the URI path /api/
are now routed to router1. And when you hit the URI http://localhost:8000/api/
, ‘/’ routing in the router 1 is executed. This is because, for router1, http://localhost:8000/api/
is considered as base path.
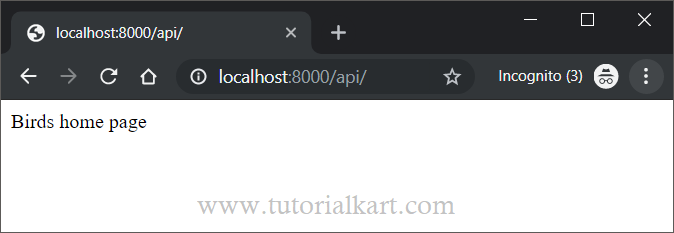
When you hit the URI http://localhost:8000/api/about/
, /about/
routing is selected.
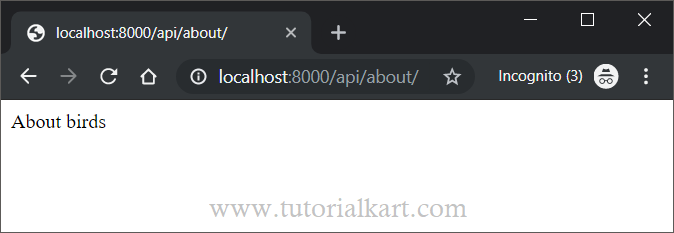
Terminal Log

Summary
When you use it for the first time, it could confuse you a little bit with the execution flow. But with practice, it can become a powerful tool to create modular express applications.