Node.js – Connect to MySQL Database
In this tutorial, we shall learn to Connect to MySQL database in Node.js using mysql.createConnection
method with an example Node.js program.
Node.js provides a module to connect to MySQL database and perform MySQL operations.
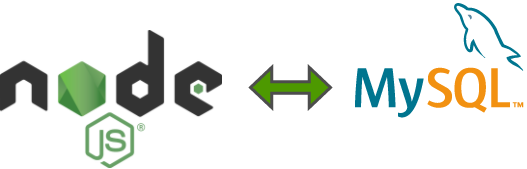
Steps to Connect to MySQL Database via Node.js
Following is a step-by-step guide to connect to MySQL database with an example.
Step 1: Make sure that MySQL is configured properly. Collect the information about IP Address, User Name and Password.
Step 2: In your node.js program, include mysql module.
var mysql = require(‘mysql‘);
MySQL module has to be installed before it can be used. Otherwise you would get an error.
To install mysql module, refer how to install MySQL in Node.js.
Step 3: Create a connection variable with IP Address, User Name and Password collected in Step 1.
var con = mysql.createConnection({
host: "localhost", // ip address of server running mysql
user: "arjun", // user name to your mysql database
password: "password" // corresponding password
});
Step 4: Make a call to connect function using connection variable, that we created in the previous step.
con.connect(function(err) {
if (err) throw err;
console.log("Connected!");
});
Function provided as argument to connect is a callback function. After node.js has tried with connecting to MySQL Database, the callback function is called with the resulting information sent as argument to the callback function.
Example 1 – Connect to MySQL Database via Node.js
In this example, we will connect to a MySQL instance running in the localhost.
connectToMySQL.js
// include mysql module
var mysql = require('mysql');
// create a connection variable with the details required
var con = mysql.createConnection({
host: "localhost", // ip address of server running mysql
user: "arjun", // user name to your mysql database
password: "password" // corresponding password
});
// connect to the database.
con.connect(function(err) {
if (err) throw err;
console.log("Connected!");
});
You may change the host as per your requirements. It could be an IP, or a URL, etc.
Open a terminal or command prompt and run this script using node command as shown in the following.
Output
$ node connectToMySQL.js
Connected!
Conclusion
In this Node.js Tutorial – Node.js MySQL – Connect to Database, we have learnt to Connect to MySQL database using Node.js MySQL Module -> createConnection method with the help of an example Node.js program.