Node.js MySQL is one of the external libraries of Node.js. It helps Node.js developers to connect to MySQL database and execute MySQL Queries.
We shall learn to install MySQL module in Node.js using npm, and use SQL statements with clearly illustrated examples.
Node.js MySQL
Following is quick view of topics we are going to learn about Node.js MySQL
- Install Node.js MySQL module
- Node.js MySQL Connect Database
- MySQL Queries in Node.js
- MySQL SELECT FROM Table Query
- MySQL INSERT INTO Table Query – Node.js MySQL Tutorial to insert records into Table
- MySQL WHERE clause with SELECT Query
- MySQL ORDER BY clause with SELECT Query– Node.js MySQL Tutorial to arrange records in an order based on one of the properties of records in table.
- MySQL UPDATE Table Query
- MySQL DELETE Records Query
- Usage of MySQL Result Object in callback function
- Usage of MySQL Fields Object in callback function
- Usage of MySQL Error Object in callback function
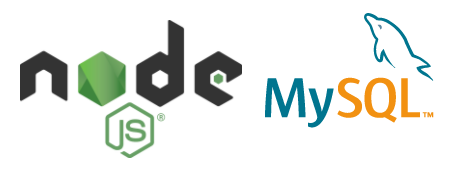
Install MySQL in Node.js
As Node.js MySQL is an external module, it can be installed using NPM (Node Package Manager).
Run the following command in terminal or command prompt to install MySQL module and use it in Node.js programs.
$ npm install mysql
After successful install, you may use MySQL module in node.js programs by declaring its usage with a require statement as shown below.
var mysql = require('mysql');
Note – If MySQL module is not installed, but used in Node.js programs, you might get the Error : Cannot find module ‘mysql’.
Create Connection to MySQL Database
To create a connection variable with IP Address (of server where MySQL server is running), User Name and Password (of user that has access the MySQL database). An example is provided below :
var con = mysql.createConnection({
host: "localhost", // ip address of server running mysql
user: "arjun", // user name to your mysql database
password: "password", // corresponding password
database: "studentsDB" // use this database to querying context
});
SELECT FROM Query
MySQL SELECT Query is used to select some of the records (with some of their properties if required) of a table.
con.query("SELECT * FROM studentsDB.students", function (err, result, fields) {
// if any error while executing above query, throw error
if (err) throw err;
// if there is no error, you have the result
console.log(result);
});
Conclusion
In this Node.js Tutorial, we have learnt to install MySQL module/package in Node.js using npm.