Modules in Node.js
A Node.js Module is a library of functions that could be used in a Node.js file.
In this tutorial, we will learn what types of modules are present in Node.js, how to import a module, and how to call a function of a module after importing.
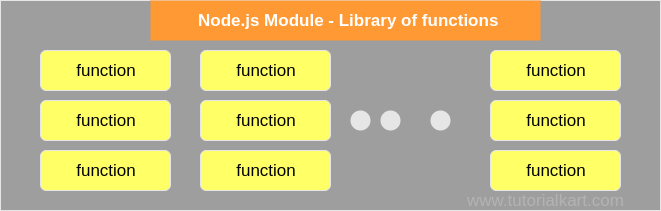
There are three types of modules in Node.js based on their location to access. They are :
Built-in Modules
These are the modules that come along with Node.js installation.
Reference to the list of Node.js Built-in Modules.
User defined Modules
These are the modules written by user or a third party.
We shall learn more in detail about user defined modules in the Node.js User Defined Modules section.
- Create a Node.js module
- Extend a Node.js module
Third party Modules
There are many modules available online which could be used in Node.js. Node Package Manager (NPM) helps to install those modules, extend them if necessary and publish them to repositories like Github for access to distributed machines.
- Install a Node.js module using NPM
- Extend a Node.js module
- Publish a Node.js module to Github using NPM
Include a module
Including a module in the Node.js files allows us to use the functions exposed by the module.
Syntax
Following is the syntax to include a module in a Node.js file.
var http = require('<module_name>');
Example
To include ‘http’ module in a Node.js file, we need to write the below require statement before using the http module.
var http = require('http');
Using Function of a Module
Once you have included a module by assigning it to a variable, the functions in the module could be accessed through the variable.
In the above module section, an example for including http module is provided. Now we shall use a function of http module called createServer() to demonstrate on how to use a function of a module.
example.js
var http = require('http'); http.createServer(function (req, res) { res.writeHead(200, {'Content-Type': 'text/plain'}); res.write('Node.js says Hello!'); res.end(); }).listen(8080);
The function creates an HTTP Server that responds with ‘Node.js says Hello!’ when an http request is made to port 8080.
Conclusion
In this Node.js Tutorial, we have learnt about Node.js Modules, how to include them in a Node.js file, and how to use a function of a Node.js Module.