Node.js Parse URL
Node.js Parse URL : In this tutorial, we shall learn how to parse URL in Node.js or split a URL into readable parts and extract search parameters using built-in Node.js URL module.
To parse URL in Node.js : use url module, and with the help of parse and query functions, you can extract all the components of URL.
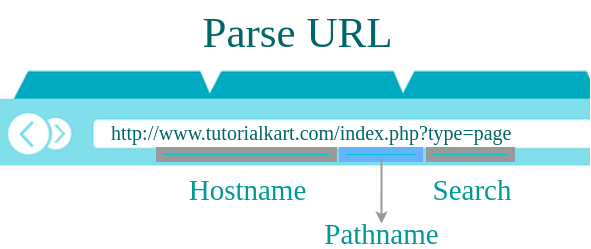
Steps – Parse URL components in Node.js
Following is a step-by-step guide to program on how to parse URL into readable parts in Node.js.
Step 1: Include URL module
var url = require('url');
Step 2: Take URL to a variable
Following is a sample URL that we shall parse.
var address = 'http://localhost:8080/index.php?type=page&action=update&id=5221';
Step 3: Parse URL using parse function.
var q = url.parse(address, true);
Step 4: Extract HOST, PATHNAME and SEARCH string using dot operator.
q.host
q.pathname
q.search
Step 5: Parse URL Search Parameters using query function.
var qdata = q.query;
Step 6: Access Search Parameters
qdata.type
qdata.action
qdata.id
Example 1 – Parse URL in Node.js
In this example, we will take a URL, and parse it into readable parts using url module.
urlParsingExample.js
// include url module
var url = require('url');
var address = 'http://localhost:8080/index.php?type=page&action=update&id=5221';
var q = url.parse(address, true);
console.log(q.host); //returns 'localhost:8080'
console.log(q.pathname); //returns '/index.php'
console.log(q.search); //returns '?type=page&action=update&id=5221'
var qdata = q.query; // returns an object: { type: page, action: 'update',id='5221' }
console.log(qdata.type); //returns 'page'
console.log(qdata.action); //returns 'update'
console.log(qdata.id); //returns '5221'
Output
$ node urlParsingExample.js
localhost:8080
/index.php
?type=page&action=update&id=5221
page
update
5221
Conclusion
In this Node.js Tutorial – Parse URL, we have learnt how to parse or split a URL into readable parts in Node.js using built-in Node.js URL module. And extract host, pathname, search and search parameters.