Create HTTP Web Server in Node.js
In this tutorial, we shall learn to create HTTP Web Server in Node.js using http.createServer() method of HTTP Built-in Module.
Node.js provides built-in module, HTTP, which is stable and is compatible with NPM ecosystem.
Steps – Create HTTP Web Server
Following is a step by step tutorial, to Create HTTP Web Server in Node.js.
Step 1 : Include HTTP Module
Create a .js file with name httpWebServer.js and open in a text editor.
Include the Built-in Node.js module, HTTP, using require function as shown below.
var http = require('http');
Step 2 : Create Server
Create a server using the method createServer() to listen at port numbered 9000.
// include http module in the file var http = require('http'); // create a server http.createServer(function (req, res) { // code to feed or request and prepare response }).listen(9000); //the server object listens on port 9000
Step 3 : Prepare response
We shall prepare a response with HTTP header and a message.
// include http module in the file var http = require('http'); // create a server http.createServer(function (req, res) { // http header // 200 - is the OK message // to respond with html content, 'Content-Type' should be 'text/html' res.writeHead(200, {'Content-Type': 'text/html'}); res.write('Node.js says hello!'); //write a response to the client res.end(); //end the response }).listen(9000); //the server object listens on port 9000
Step 4 : Run the Web Server
Run the httpWebServer.js file (from previous step) to create and make the server listen at port 9000.
$ node httpWebServer.js
The server will be up and running.
Step 5 : Test the Web Server
Open a browser and hit the url, “http://127.0.0.1:9000/”, to trigger a request to our Web Server.
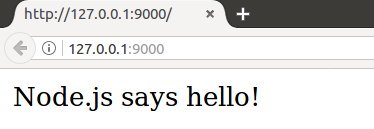
Voila! We have created a HTTP Web Server that listens on port numbered 9000 and responds with HTML formatted text message ”Node.js says hello!” for any request.
This may not be a full fledged Web Server that you expect for your projects, but this is for sure a stepping in our journey of building HTTP Web Server.
Conclusion
In this Node.js Tutorial – Create HTTP Web Server in Node.js, we have used http.createServer() method of HTTP Built-in Node.js module to create HTTP Web Server that responds to the requests made at a port.