Node.js Append to a File
To append data to file in Node.js, use Node FS appendFile() function for asynchronous file operation or Node FS appendFileSync() function for synchronous file operation.
In this tutorial, we shall learn
- Syntax of appendFile() function
- Syntax of appendFileSync() function
- Example for appendFile() : Appending data to file Asynchronously
- Example for appendFileSync() : Appending data to file Synchronously
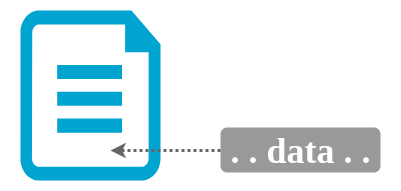
Syntax – appendFile()
The syntax of appendFile() function is
fs.appendFile(filepath, data, options, callback_function);
Callback function is mandatory and is called when appending data to file is completed.
Syntax – appendFileSync()
The syntax of appendFileSync() function is
fs.appendFileSync(filepath, data, options);
where :
- filepath [mandatory] is a String that specifies file path
- data [mandatory] is what you append to the file
- options [optional] to specify encoding/mode/flag
Note : If file specified does not exist, a new file is created with the name provided, and data is appended to the file.
Example 1 – Node.js Append data to file asynchronously using appendFile()
To append data to a file asynchronously in Node.js, use appendFile() function of Node FS as shown below.
nodejs-append-to-file-example.js
// Example Node.js program to append data to file var fs = require('fs'); var data = "\nLearn Node.js with the help of well built Node.js Tutorial."; // append data to file fs.appendFile('sample.txt',data, 'utf8', // callback function function(err) { if (err) throw err; // if no error console.log("Data is appended to file successfully.") });
Output
arjun@arjun-VPCEH26EN:~/nodejs$ node nodejs-append-to-file-example.js Data is appended to file successfully.
File before appending
Welcome to www.tutorialkart.com.
File after appending
Welcome to www.tutorialkart.com. Learn Node.js with the help of well built Node.js Tutorial.
Example 2 – Node.js Append data to file synchronously using appendFileSyc()
To append data to a file synchronously in Node.js, use appendFileSync() function of Node FS as shown below.
nodejs-append-to-file-example-2.js
// Example Node.js program to append data to file var fs = require('fs'); var data = "\nLearn Node.js with the help of well built Node.js Tutorial."; // append data to file fs.appendFileSync('sample.txt',data, 'utf8'); console.log("Data is appended to file successfully.")
Output
arjun@arjun-VPCEH26EN:~/nodejs$ node nodejs-append-to-file-example-2.js Data is appended to file successfully.
File before appending
Welcome to www.tutorialkart.com.
File after appending
Welcome to www.tutorialkart.com. Learn Node.js with the help of well built Node.js Tutorial.
Conclusion
In this Node.js Tutorial – Node.js Append to a File, we have learnt to append data to a file in Node.js, synchronously and asynchronously using appendFileSync() and appendFile() functions of Node FS respectively with Example Node.js programs.