Express.js Routes
An Express.js Route defines how our Express application can respond to a client request to with a specific URI (or path) and a specific HTTP request method (GET, POST, etc.).
To understand the need of an Express.js Route, let us dive into an example.
Create a basic Express application as shown beow.
app.js
var express = require('express')
var app = express()
// start the server
var server = app.listen(8000, function(){
console.log('Listening on port 8000...')
})
All we have done is, instantiate an express application, started it at port 8000. Now, open a browser and hit the url http://localhost:8000/
.
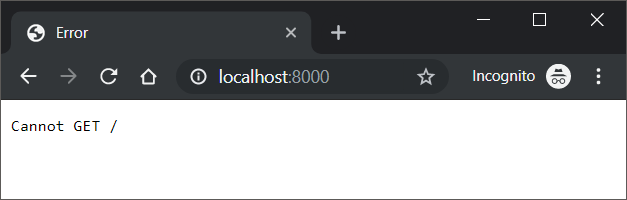
The reponse is that it cannot GET the resource ‘/’.
Even there is no error in the console. The app is running fine.
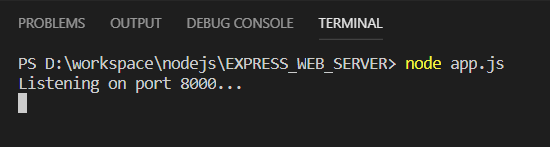
Why is that? Because, in our Express application, we started the server, but did not define what has to happen when a request hits the server.
This is where Express.js Routes come into picture. Following is a simple express route.
app.get('/', function (req, res) {
res.send('This is a basic Example for Express.js by TUTORIALKART')
})
What does this route define? This route defines that, execute the statements inside the function, when you receive a GET
request with request url as /
.
In the following screenshot is a route, where the function is executed for a GET request with request url /hello/
.
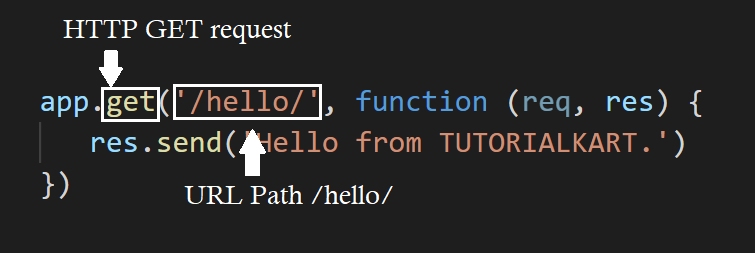
Let us define some routes in our app.js and start the server.
app.js
var express = require('express')
var app = express()
// route that gets executed for GET request and the request url path '/' or root
app.get('/', function (req, res) {
res.send('Home.')
})
// route that gets executed for GET request and the request url path '/hello/'
app.get('/hello/', function (req, res) {
res.send('Hello page.')
})
// route that gets executed for GET request and the request url path '/bye/'
app.get('/bye/', function (req, res) {
res.send('Bye page.')
})
// start the server
var server = app.listen(8000, function(){
console.log('Listening on port 8000...')
})
Start the express application..
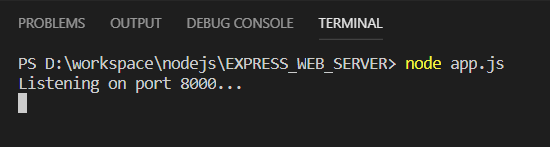
Now hit the urls in the browser. By default, browser sends a GET request.
GET request with URL path http://localhost:8000/
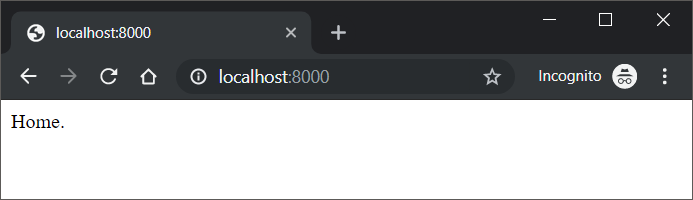
GET request with URL path http://localhost:8000/hello/
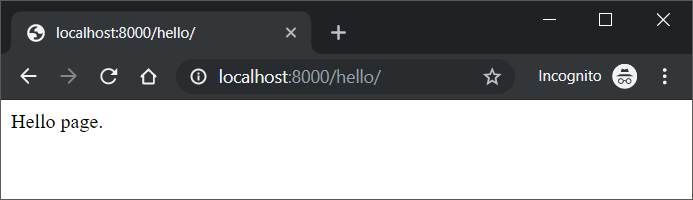
GET request with URL path http://localhost:8000/bye/
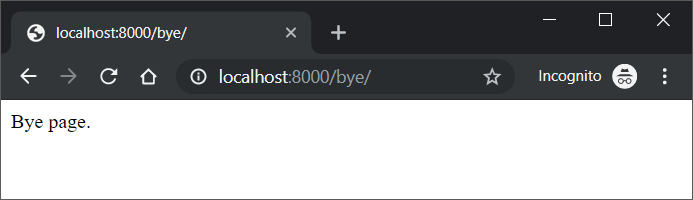
Express route with multiple functions
You can provide one or more functions in the route. Each function is called a middleware. [Reference: Express.js Middleware]
app.js
var express = require('express')
var app = express()
// express route with multiple functions
app.get('/hello/', function (req, res, next) {
res.write('Hello page. ')
next()
}, function(req, res, next){
res.write('Hello again. ')
res.end()
})
// start the server
var server = app.listen(8000, function(){
console.log('Listening on port 8000...')
})
And in the browser, output is
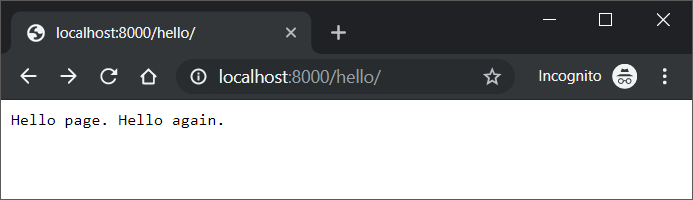
You can also define these functions separately for modularity as shown below.
var express = require('express')
var app = express()
function hello(req, res, next) {
res.write('Hello page. ')
next()
}
function helloagain(req, res, next){
res.write('Hello again. ')
res.end()
}
// express route with multiple functions
app.get('/hello/', hello, helloagain)
// start the server
var server = app.listen(8000, function(){
console.log('Listening on port 8000...')
})
Summary
In this Express.js Tutorial, we have learned what an Express.js Route is, how to define Express.js Route, and how to use these Routes to serve different kinds of HTTP methods against different URL paths.