Basic Kotlin Program – Hello World
In our previous tutorial, we have learned to create a Kotlin Project in IntelliJ IDEA. In this tutorial, we shall write a basic Kotlin program and run it. Also, a detailed discussion about the typical structure of a Kotlin program and components present in it are provided.
Kotlin supports procedural programming in addition to Object-Oriented Programming(Classes and Methods). Using the following basic Kotlin program example, we shall make some useful observations.
Example – Kotlin Program
Consider the following Kotlin program
HelloWorld.kt
/** * Kotlin Program */ fun main(args: Array<String>) { println("Hello World!") }
Run the above program. If you are using IntelliJ IDEA, you may right click on the code and click on ‘Run’ menu item as shown below.
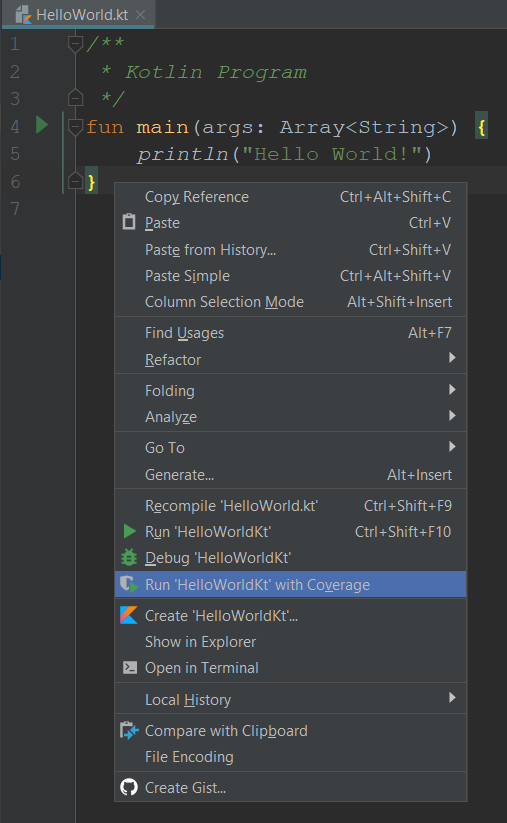
When the program is run, you will get the following output printed in the Run window.
Output
Hello World!
Let us together make out some observations from the above three lines of Kotlin code:
Kotlin File
We write the whole program in a Kotlin File. The extension of this file is .kt
. You can run this Kotlin program in your IDE, once the Kotlin setup in your IDE is successful.
Kotlin Comments
/** * Kotlin Program */
The first three lines in the HelloWorld.kt, is a multiple line comment. Comments are ignored for execution.
Main Function
fun main( ) { }
The keyword “fun” is used to define a function. You can have multiple functions in a Kotlin program.
We have defined only one function, i.e., “main” in HelloWorld.kt.
Since main is the entry point for a Kotlin program, the statements inside the main function are executed, when you run this Hello World Kotlin program.
Function Arguments
(args: Array<String>)
The entry “main” function can accept Array of Strings as an input (arguments) to the function, which are the inputs to the Kotlin program.
The argument’s type is specified after the variable name separated by a colon.
Function Body
{ }
The function body is enclosed in curly brackets.
There is no semicolon at the end of println()
statement to denote the end of a statement. Kotlin compiler can make out the end of a statement with a new line or semicolon. So, the semicolon is an optional means of ending a statement in Kotlin.
Conclusion
This is just an introduction to the basic Hello World program in Kotlin, and the different concepts used to create this program. Going forward, we shall go through the above discussed, and many other concepts in detail.
Concluding this Kotlin Tutorial – Basic Kotlin Program Example, we have learned about the basic building blocks and structure of a Kotlin Program. In our next tutorial, we shall look into different ways of converting Java Files to Kotlin Files.