In this tutorial, you shall learn how to read the contents of a file character by character in Kotlin, using FileReader class, with example programs.
Kotlin – Read a file character by character
To read a file character by character in Kotlin, we can use read() function from the java.io.FileReader class.
Steps to read a file character by character
- Consider that we are given a file identified by a path.
- Create a FileReader object from the given path to file.
- Call read() function on the file object as long as the returned character is not a null character code
-1
. - FileReader.read() function reads the next character in the file, starting from the beginning. So, the first call to read() function returns the first character, the second call to the function returns the second character, and so on.
</>
Copy
val file = File("path/to/file")
val reader = FileReader(file)
var char: Int? = reader.read()
while (char != -1) {
val c = char?.toChar()
char = reader.read()
}
Example
In the following program, we read the text file info.txt
character by character, and print these characters to standard output.
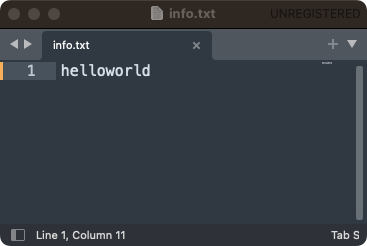
Main.kt
</>
Copy
import java.io.FileReader
fun main() {
val filename = "info.txt"
val reader = FileReader(filename)
var char: Int? = reader.read()
while (char != -1) {
val c = char?.toChar()
println(c)
char = reader.read()
}
reader.close()
}
Output
h
e
l
l
o
w
o
r
l
d
Conclusion
In this Kotlin Tutorial, we learned how to read a file character by character using java.io.FileReader.read() function.