In this tutorial, you shall learn how to move a file from one location to another in Kotlin using renameTo() function, with example programs.
Kotlin – Move a File
To move a file in Kotlin, you can use renameTo() function of java.io.File class.
You may wonder how renaming a file moves the file. In this case, we do not rename the actual file name, but the file path. We change the directory path of the file. Therefore, when you rename using renameTo(), the file is moved to the target path.
Steps to move a file
- Consider that we need to move a file from one location (source) to another location (target).
- Create two file objects: one for the source file, and one for the target file, with respective paths.
- Call renameTo() function on the source file object and pass the target file object as argument. The function returns true if renaming is successful, else it returns false. And the source file is moved to the target location.
val sourceFile = File("source/file")
val targetFile = File("target/file")
sourceFile.renameTo(targetFile)
Example
Consider that we have a file mydata/set1/info.txt
, and we would like to move it to mydata/set2/info.txt
.
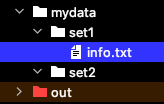
Here, we have not changed the file name (but you may) in target file path. But, we have changed its parent directory. Therefore, the renaming operation moves the file info.txt
from mydata/set1
to mydata/set2
.
Main.kt
import java.io.File
fun main() {
val sourceFile = File("mydata/set1/info.txt")
val targetFile = File("mydata/set2/info.txt")
if ( sourceFile.renameTo(targetFile) ) {
println("File copied successfully.")
} else {
println("Could not copy file.")
}
}
Output
File copied successfully.
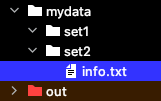
Reference tutorials for the above program
Conclusion
In this Kotlin Tutorial, we learned how to copy a file using java.io.File.renameTo() function.