In this tutorial, you shall learn how to rename a directory in Kotlin identified by a given path, using File.renameTo() function, with example programs.
Kotlin – Rename a Directory
To rename a directory in Kotlin, you can use renameTo() function of java.io.File class.
Steps to rename a directory
- Consider that we have a directory (source) given by a path, and have to rename this directory to a new name (target).
- Create two file objects: one for the source directory, and one for the target directory (the new name to which we would like to rename to), with respective paths.
- Call renameTo() function on the source directory and pass the target directory as argument. The function returns true if renaming the directory is successful, else it returns false.
</>
Copy
val sourceDir = File("path/to/source")
val targetDir = File("path/to/target")
sourceDir.renameTo(targetDir)
Example
Consider that we have a directory part1
at mydata/set1/
, and we would like to rename it to part1_foo
.
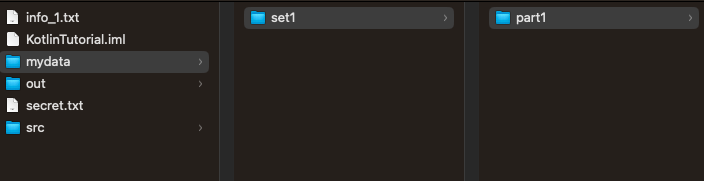
In the following program, we shall rename the directory mydata/set1/part1
to mydata/set1/part1_foo
by using File.renameTo() function.
Main.kt
</>
Copy
import java.io.File
fun main() {
val sourceDir = File("mydata/set1/part1")
val targetDir = File("mydata/set1/part1_foo")
if ( sourceDir.renameTo(targetDir) ) {
println("Directory renamed successfully.")
} else {
println("Could not rename directory.")
}
}
Output
Directory renamed successfully.
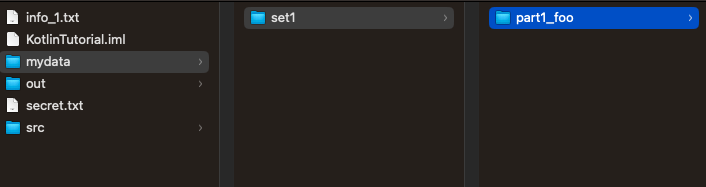
Reference tutorials for the above program
Conclusion
In this Kotlin Tutorial, we learned how to rename a directory using java.io.File.renameTo() function.