In this tutorial, you shall learn how to replace all the occurrences of a specific string in a file in Kotlin, with example programs.
Kotlin – Replace string in a file
To replace a specific string in a file in Kotlin, you can read the file content to a string, then replace all the occurrences of specified search string with a replacement string, and write the resulting content back to the file.
Steps to replace string in a file
- Consider that we have a text file identified by a given path. We have to replace all the occurrences of
oldString
withnewString
in this text file. - Create a file object with the given path using java.io.File class.
- Read content from the file object using File.readText() function
- Replace the old string
oldString
withnewString
using String.replace() function - Write the resulting string back to file using File.writeText() function.
val file = File("path/to/text/file") val content = file.readText() val updatedContent = content.replace(oldString, newString) file.writeText(updatedContent)
ADVERTISEMENT
Example
For this example, we will consider a file named info.txt.
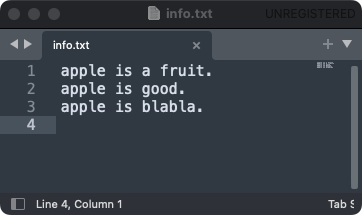
In the following program, we will replace the search string "apple"
with the replacement string "mango"
, in the file info.txt.
Main.kt
import java.io.File fun main() { val filePath = "info.txt" val oldString = "apple" val newString = "mango" val file = File(filePath) val content = file.readText() val updatedContent = content.replace(oldString, newString) println("$oldString replaced with $newString in $filePath") file.writeText(updatedContent) }
Output
apple replaced with mango in info.txt
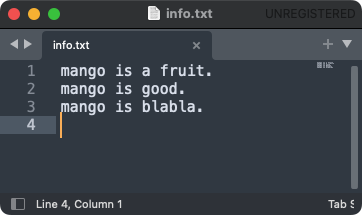
Reference tutorials for the above program
Conclusion
In this Kotlin Tutorial, we learned how to replace specific search string in a file by a replacement string.