In this tutorial, you shall learn how to create a directory recursively in Kotlin, using File.mkdirs() function, with example programs.
Kotlin – Create a directory recursively
It is a scenario in which we are given a path of a directory to be created, but its parent directories are not present. In that case, we may need to create the parent directories first, before creating the final directory.
This is when we use mkdirs() function of java.io.File class.
mkdirs() function creates the directory defined by given path, including any parent directories that are not present. The function returns a boolean value of true if the directory is created successfully, else it returns false.
Steps to create a directory recursively
- Consider that we are given a path to a directory.
- Create a file object with the given path to directory using File() class.
- Call mkdirs() function on the file object.
val directory = File("path/to/directory")
directory.mkdirs()
Examples
1. Create a new directory recursively
In the following program, we create a directory defined by the path mydata/set1/part1
.
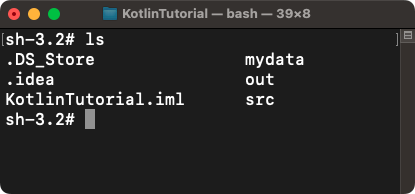
We have mydata
directory. We need to create part1
directory. But the parent of part1
, i.e., set1
is not already present.
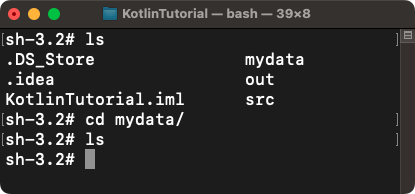
When we use mkdirs() function, the parent directory that does not exist must be created.
Main.kt
import java.io.File
fun main() {
val directory = File("mydata/set1/part1")
if (directory.mkdirs()) {
println("Directory created successfully.")
} else {
println("Directory already present, or some failure.")
}
}
Output
Directory created successfully.
2. Directory already present
Now, let us run the program again. Since the directory is already present, mkdirs() function returns false, and else-block is executed.
Main.kt
import java.io.File
fun main() {
val directory = File("mydata/set1/part1")
if (directory.mkdirs()) {
println("Directory created successfully.")
} else {
println("Directory already present, or some failure.")
}
}
Output
Directory already present, or some failure.
Conclusion
In this Kotlin Tutorial, we learned how to create a new directory recursively using File.mkdirs() function.