In this tutorial, you shall learn how to check if a given file is readable in Kotlin, using File.canRead() function, with example programs.
Kotlin – Check if file is readable
To check if given file is readable in Kotlin, we can use canRead() function from the java.io.File class.
Steps to check if file is readable
- Consider that we are given a file identified by a path.
- Create a file object from the given path to file. Call canRead() function on the file object. The function returns true if the file is readable, or false if the file is not readable.
val file = File("path/to/file") file.canRead()
Examples
1. Check if file “info.txt” is readable
In the following program, we check if the text file: info.txt
is readable.
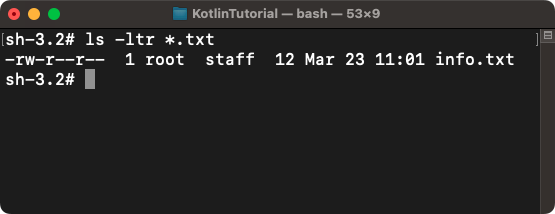
From the permissions information, you can observe that the file has read access for all users.
Main.kt
import java.io.File fun main() { val file = File("info.txt") if (file.canRead()) { println("$file is readable") } else { println("$file is not readable") } }
Output
info.txt is readable
2. Check if file “secret.txt” is readable
In the following program, we check if the text file: secret.txt
is readable.
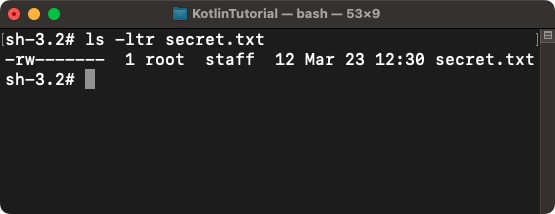
From the permissions information, you can observe that the file has read access only to root user.
Main.kt
import java.io.File fun main() { val file = File("secret.txt") if (file.canRead()) { println("$file is readable") } else { println("$file is not readable") } }
Output
secret.txt is not readable
The file is not readable for the user that accessed the file from Kotlin program.
Conclusion
In this Kotlin Tutorial, we learned how to check if file is readable using java.io.File.canRead() function.