In this tutorial, you shall learn how to read the contents of a file to a string in Kotlin, using File.readText() function, with example programs.
Kotlin – Read file into a string
To read the complete contents of a file into a string in Kotlin, you can use readText() function of java.io.File class.
Steps to read a file into a string
- Consider that we are given a file identified by a path.
- Create a file object from the given file path, and call readText() function on the file object. The function returns the contents of given file as a string.
val file = File("path/to/file") file.readText()
ADVERTISEMENT
Example
In the following program, we read the contents of the text file info.txt
into a string, and print this string to standard output.
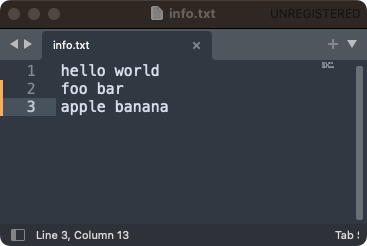
Main.kt
import java.io.File fun main() { val file = File("info.txt") val text = file.readText() println(text) }
Output
hello world foo bar apple banana
Conclusion
In this Kotlin Tutorial, we learned how to read contents a file into a string using java.io.File.readText() function.