In this tutorial, you shall learn how to read the contents of a file line by line in Kotlin, using Scanner.nextLine() function, with example programs.
Kotlin – Read a file line by line
To read a file line by line in Kotlin, we can use Scanner class.
Steps to read a file line by line
- Consider that we are given a file identified by a path.
- Create a file object from the given file path, and create a Scanner object from the file object.
- Using Scanner.hasNextLine() and Scanner.nextLine() functions, we can read the file contents line by line.
</>
Copy
val file = File("path/to/file")
val sc = Scanner(file)
while (sc.hasNextLine()) {
val line = sc.nextLine()
println(line)
}
Example
In the following program, we read the text file info.txt
line by, and print these lines to standard output.
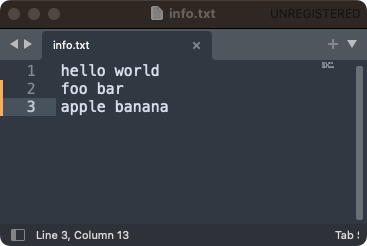
Main.kt
</>
Copy
import java.io.File
import java.io.IOException
import java.util.*
fun main() {
val fileName = "info.txt"
try {
val file = File(fileName)
val sc = Scanner(file)
while (sc.hasNextLine()) {
val line = sc.nextLine()
println(line)
}
} catch (e: IOException) {
e.printStackTrace()
}
}
Output
hello world
foo bar
apple banana
Conclusion
In this Kotlin Tutorial, we learned how to read a file line by line using java.util.Scanner class.