In this tutorial, you shall learn how to move a directory from one location to another in Kotlin using renameTo() function, in a step by step process, with example programs.
Kotlin – Move a Directory
To move a directory in Kotlin, you can use renameTo() function of java.io.File class.
You may wonder how renaming a directory moves the directory. In this case, we do not rename the actual directory name, but the directory path. We change the path of the directory such that the target location is some other folder. Therefore, when you rename using renameTo(), the directory is moved to the target path.
Steps to move a directory
- Consider that we would like to move a directory from source location to target location.
- Create two file objects: one for the source directory, and one for the target directory, with respective paths.
- Call renameTo() function on the source directory file object and pass the target directory file object as argument. The function returns true if renaming is successful, else it returns false. And the source directory is moved to the target location.
val sourceDir = File("source/directory") val targetDir = File("target/directory") sourceDir.renameTo(targetDir)
Example
Consider that we have a directory mydata/set1/part1
, and we would like to move it to mydata/set2/part1
.
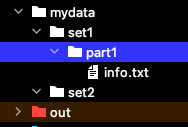
Here, we have not changed the directory name (but you may) in target file path. But, we have changed its parent directory. Therefore, the renaming operation moves the directory part1
from mydata/set1
to mydata/set2
.
Main.kt
import java.io.File fun main() { val sourceDir = File("mydata/set1/part1") val targetDir = File("mydata/set2/part1") if ( sourceDir.renameTo(targetDir) ) { println("Directory copied successfully.") } else { println("Could not copy directory.") } }
Output
Directory copied successfully.
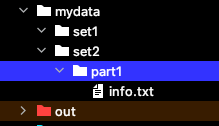
Reference tutorials for the above program
Conclusion
In this Kotlin Tutorial, we learned how to copy a directory using java.io.File.renameTo() function.