In this tutorial, you shall learn how to remove all the content in a file in Kotlin using File.writeText() function, with example programs.
Kotlin – Remove all the content in a file
To remove all the content from a text file in Kotlin, you can overwrite the file with an empty string.
Steps to remove the content in a file
- Consider that we have a text file identified by a given path.
- Create a file object with the given path using java.io.File class, and write an empty string to file using File.writeText() function.
</>
Copy
val file = File("path/to/text/file")
file.writeText("")
Example
For this example, we will consider a file named info.txt.
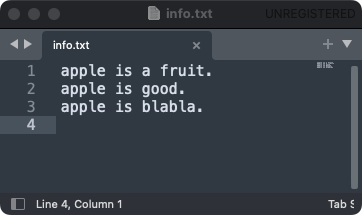
In the following program, we will remove all the content from the file info.txt, and make it empty.
Main.kt
</>
Copy
import java.io.File
fun main() {
val filePath = "info.txt"
val file = File(filePath)
file.writeText("")
println("$filePath emptied successfully.")
}
Output
info.txt emptied successfully.
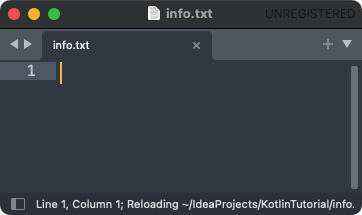
Reference tutorials for the above program
Conclusion
In this Kotlin Tutorial, we learned how to remove all the content from a text file using File.writeText() function.