In this tutorial, you will learn how to read a file as InputStream in Java, using FileInputStream class and InputStream class of java.io.
Java – Read file as InputStream
To read a file as InputStream in Java,
- Given a file path, create a new instance of FileInputStream using the given file path, and assign it to an InputStream type object.
- Read the input stream byte by byte using a Java While loop.
If filePath
is the given file path, then use the following statement to get the InputStream.
InputStream inputStream = new FileInputStream(filePath)
1. Read the file “data.txt” as InputStream in Java
In the following program, we take a file path "/Users/tutorialkart/data.txt"
, read the content of this file using InputStream, and print the bytes of file one by one to standard output.
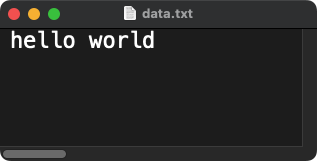
In the program,
- Given a file path filePath as String.
String filePath = "/Users/tutorialkart/data.txt";
- Use Java’s try with resource syntax to create the InputStream.
- Create an instance of InputStream using FileInputStream() class. Store the InputStream object in a variable, say inputStream.
try (InputStream inputStream = new FileInputStream(filePath)) { }
- Inside the try block, read the content from inputStream byte by byte using While loop. Typecast the byte to char, and print it to output.
int byteRead; while ((byteRead = inputStream.read()) != -1) { System.out.print((char) byteRead); }
- If there could be an IOException while handling the file, catch the IOException and address it in a catch block.
try( ) { } catch (IOException e) { e.printStackTrace(); }
The following is the complete Java program, to read the file as InputStream, and then print the content of the InputStream.
Java Program
import java.io.FileInputStream; import java.io.IOException; import java.io.InputStream; public class Main { public static void main(String[] args) { String filePath = "/Users/tutorialkart/data.txt"; try (InputStream inputStream = new FileInputStream(filePath)) { int byteRead; while ((byteRead = inputStream.read()) != -1) { System.out.print((char) byteRead); } } catch (IOException e) { e.printStackTrace(); } } }
Output
hello world
ADVERTISEMENT
Conclusion
In this Java File Operations tutorial, we learned how to read the file as InputStream using java.io.FileInputStream class, with examples.