Java System.setIn() – Examples
In this tutorial, we will learn about the Java System.setIn() function, and learn how to use this function to set the standard input stream, with the help of examples.
setIn(InputStream in)
System.setIn() reassigns the “standard” input stream with specified InputStream object.
By default, Console input is the standard input stream. But, using setIn(), you can set the standard input stream to a InputStream object or object of any subclass of InputStream.
Syntax
The syntax of setIn() function is
setIn(InputStream in)
where
Parameter | Description |
---|---|
in | The new standard input stream for System. |
Returns
The function returns void.
Example 1 – setIn()
In this example, we will set a FileInputStream object as the System standard input using setIn(). System can read the input from this input stream.
We will use System.in.readAllBytes() to read the data from this input stream.
The following file input.txt
is used to create FileInputStream
object which we have passed as argument to setIn() method.
input.txt
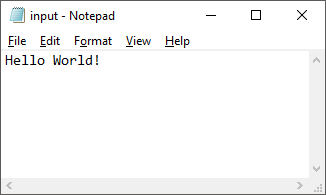
Java Program
import java.io.FileNotFoundException; import java.io.IOException; import java.io.FileInputStream; public class Example { public static void main(String[] args) { try { System.setIn(new FileInputStream("input.txt")); String content = new String( System.in.readAllBytes() ); System.out.println(content); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } } }
Output
Hello World!
Example 2 – setIn() – Null InputStream
In this example, we will set a null
value, for InputStream
object, and set this as the System standard input using setIn(). Since the argument to setIn() is null
, the method throws java.lang.NullPointerException
.
Java Program
import java.io.FileNotFoundException; import java.io.IOException; public class Example { public static void main(String[] args) { try { System.setIn(null); String content = new String( System.in.readAllBytes() ); System.out.println(content); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } } }
Output
Exception in thread "main" java.lang.NullPointerException at Example.main(Example.java:8)
Conclusion
In this Java Tutorial, we have learnt the syntax of Java System.setIn() function, and also learnt how to use this function with the help of examples.