In this tutorial, you will learn how to read a file as byte array in Java, using readAllBytes() method of java.nio.file.Files class.
Java – Read file as byte array
To read a file as byte array in Java, given a file path, call readAllBytes() method of the java.nio.file.Files class and pass the file path as argument to the method. The readAllBytes() method returns a byte array with the bytes of the file.
If filePath
is the given file path, then use the following expression to get the bytes in file.
Files.readAllBytes(filePath)
1. Read the file “data.txt” as byte array in Java
In the following program, we take a file path "/Users/tutorialkart/data.txt"
, read the content of this file as byte array, and print them to standard output.
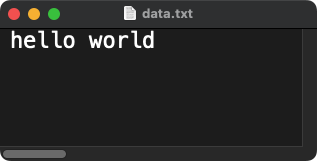
In the program,
- Create a Path object filePath from the given file path.
- Pass the file path object to readAllBytes() method of the java.nio.file.Files class.
- The readAllBytes() method returns byte array created from the content of the file. Store the returned value in a variable fileBytes.
- Iterate over the byte array using a Java For loop, and print the bytes in byte array to standard output.
- If there is IOException while handling the file, you may address it using a Java Try Catch statement.
Java Program
import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.Paths; import java.io.IOException; public class Main { public static void main(String[] args) { try { // Prepare file path Path filePath = Paths.get("/Users/tutorialkart/data.txt"); // Read bytes from the file as a byte array byte[] fileBytes = Files.readAllBytes(filePath); // Iterate over bytes and print them for (byte b : fileBytes) { System.out.print(b + " "); } } catch (IOException e) { System.out.println("An error occurred: " + e.getMessage()); } } }
Output
104 101 108 108 111 32 119 111 114 108 100 10
Conclusion
In this Java File Operations tutorial, we learned how to read the file as byte array using java.nio.file.Files readAllBytes() method, with examples.