Passwords have become lengthy and complicated for security purposes. At times, it becomes difficult to keep track of typing the characters. To facilitate the user with the display of actual characters of password when needed is a useful functionality. Many web logins and android applications are embedding this functionality for user convenience.
Show/Hide Password in Android EditText – Kotlin
In this Android Tutorial, we shall demonstrate on how to show/hide password in Android EditText with the help of an example Application.
Following is a quick glimpse of what we do for showing or hiding the password.
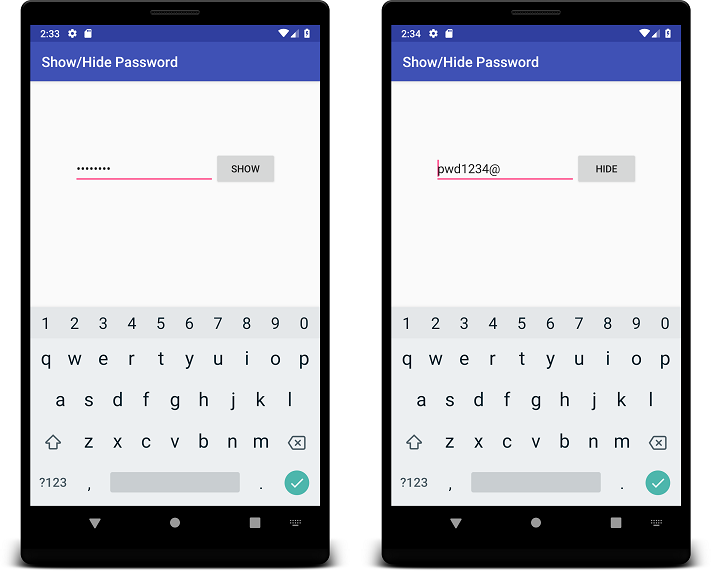
ADVERTISEMENT
Steps to show/hide password
- EditText should be provided with attribute of
android:inputType="textPassword"
. - Password can be shown or hidden using
EditText.transformationMethod
. - To show the password, set
pwd.transformationMethod = PasswordTransformationMethod.getInstance()
. - To hide the password, set
pwd.transformationMethod = HideReturnsTransformationMethod.getInstance()
.
Create an Android Project and replace the activity_main.xml and MainActivity.kt with the following code.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <LinearLayout android:orientation="horizontal" android:gravity="center" android:layout_marginTop="100sp" android:layout_width="match_parent" android:layout_height="wrap_content"> <EditText android:id="@+id/pwd" android:layout_width="wrap_content" android:layout_height="wrap_content" android:minWidth="200sp" android:hint="Password" android:inputType="textPassword" /> <Button android:id="@+id/showHideBtn" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Show"/> </LinearLayout> </LinearLayout>
MainActivity.kt
package com.tutorialkart.showhidepassword import android.support.v7.app.AppCompatActivity import android.os.Bundle import kotlinx.android.synthetic.main.activity_main.* import android.text.method.HideReturnsTransformationMethod; import android.text.method.PasswordTransformationMethod; class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) showHideBtn.setOnClickListener { if(showHideBtn.text.toString().equals("Show")){ pwd.transformationMethod = HideReturnsTransformationMethod.getInstance() showHideBtn.text = "Hide" } else{ pwd.transformationMethod = PasswordTransformationMethod.getInstance() showHideBtn.text = "Show" } } } }