Android Draw SVG to Canvas
ImageView is used to display Bitmap, Drawable or such image sources.
SVG (Scalable Vector Graphics) is used to define vector-based graphics. SVG defines the graphics in XML format and when rendered the picture do not degrade in quality if they are zoomed or resized. Although it is commonly used on web, with the advantages it has, we may render and use it on Android.
In the following screenshot, we have an Android screen displaying SVG image.
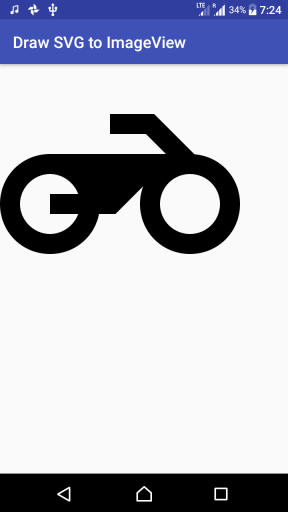
In this tutorial, we shall learn how to parse SVG file, draw it on canvas, then load Canvas’ bitmap onto a ImageView.
Following is the structure of Android Application and location of various files used.
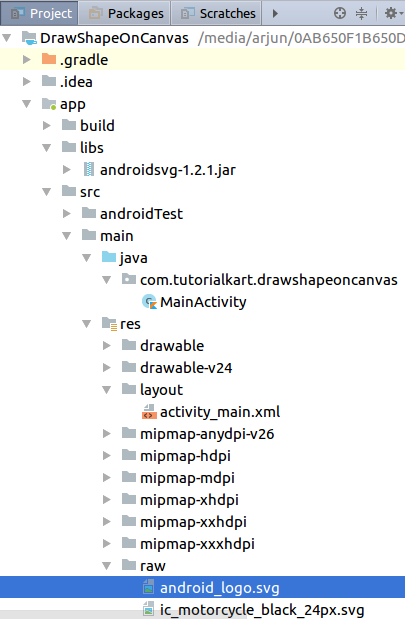
Android, by default, does not render SVG. So we shall use a third party library AndroidSVG. You may download the jar-file from AndroidSVG JAR Download. Follow Add External Jar to Library/Dependencies to add the jar file to project dependencies. Once you add the Jar file, follow the below steps.
Steps to draw SVG to Canvas
Step 1: Place the SVG file in res/raw folder.
Step 2: Read SVG resource file using SVG Class.
val svg = SVG.getFromResource(resources, R.raw.ic_motorcycle_black_24px)
Step 3: Set Width and Height (if necessary). SVG may have width and height values and if you want to overwrite those values, you may do so using SVG.documentHeight and SVG.documentWidth.
svg.documentHeight = 600F svg.documentWidth = 600F
Step 4: Create a Bitmap and a Canvas with the Bitmap.
val bitmap = Bitmap.createBitmap(700,700, Bitmap.Config.ARGB_8888) val canvas = Canvas(bitmap)
Step 5: Render SVG to Canvas.
svg.renderToCanvas(canvas)
Step 6: Use Canvas’ Bitmap to draw the Bitmap to your required View. Here we shall set the bitmap as background to a ImageView.
imageV.background = BitmapDrawable(resources, bitmap)
Example – Draw SVG to Canvas
Create an Android Application with Empty Activity and replace the content of layout and Activity files with the following.
Complete code of activity_main.xml and MainActivity.kt are provided below.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <ImageView android:id="@+id/imageV" android:gravity="center" android:layout_width="wrap_content" android:layout_height="wrap_content"/> </android.support.constraint.ConstraintLayout>
MainActivity.kt
package com.tutorialkart.drawshapeoncanvas import android.graphics.Canvas import android.support.v7.app.AppCompatActivity import android.os.Bundle import android.graphics.Bitmap import android.graphics.drawable.BitmapDrawable import com.caverock.androidsvg.SVG import kotlinx.android.synthetic.main.activity_main.* class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) // Read an SVG from the assets folder val svg = SVG.getFromResource(resources, R.raw.ic_motorcycle_black_24px) if (svg.getDocumentWidth() !== -1F) { // set your custom height and width for the svg svg.documentHeight = 600F svg.documentWidth = 600F // create a canvas to draw onto val bitmap = Bitmap.createBitmap(700,700, Bitmap.Config.ARGB_8888) val canvas = Canvas(bitmap) // canvas - white background canvas.drawARGB(0,255, 255, 255) // Render our document onto our canvas svg.renderToCanvas(canvas) // set the bitmap to imageView imageV.background = BitmapDrawable(resources, bitmap) } } }
Conclusion
In this Kotlin Android Tutorial – Draw SVG to Canvas, we have learnt to render SVG and draw it to a Canvas.