Change Button background in Kotlin Android
In this Android Tutorial, we shall learn to dynamically change button background in Kotlin Android.
There could be scenarios where you might need to change the background of a button to show a state of the application or status of an update or status of a player, etc.
setBackgroundResource() method is used to change the button background programmatically. setBackgroundResource(int id) accepts id of drawable resource and applies the background to the button.
Useful links
To set a drawable background to button : Custom design for Button background
To set a onClickListener to button : Button setOnClickListener
Example – Dynamically Change Button Background in Kotlin Android
In the following Kotlin Android Example, we shall create a button in layout xml and dynamically change its background on the button click using Button OnClickListener.
layout xml file : activity_change_button_background.xml
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.tutorialkart.myapplication.ChangeButtonBackground">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:orientation="vertical">
<Button
android:id="@+id/button_changing"
android:padding="15dp"
android:background="@drawable/btn_center_gradient"
android:textColor="#FFFFFF"
android:text="Changing Button"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</LinearLayout>
</android.support.constraint.ConstraintLayout>
Kotlin Activity file : ChangeButtonBackground.kt
package com.tutorialkart.myapplication
import android.support.v7.app.AppCompatActivity
import android.os.Bundle
import android.widget.Button
class ChangeButtonBackground : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_change_button_background)
var button_background : Int = 1;
var button_change = findViewById(R.id.button_changing) as Button;
button_change.setOnClickListener {
if(button_background==2){
button_change.setBackgroundResource(R.drawable.btn_center_gradient);
button_background=1;
} else if(button_background==1){
button_change.setBackgroundResource(R.drawable.btn_oval_gradient);
button_background=2;
}
}
}
}
/app/src/main/res/drawable/btn_center_gradient.xml
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle">
<gradient android:startColor="#44e3ff"
android:centerColor="#12434c"
android:endColor="#258191"
android:angle="90" />
</shape>
/app/src/main/res/drawable/btn_oval_gradient.xml
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="oval">
<gradient android:startColor="#ffc456"
android:centerColor="#7a5d28"
android:endColor="#ad853a"
android:angle="90"
/>
</shape>
Following are the screenshots of the application.
Click on the button to change the background.
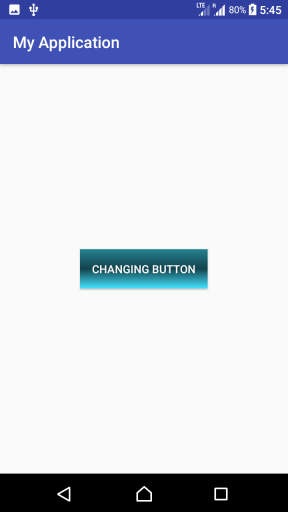
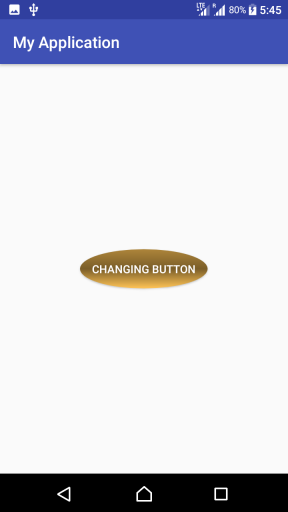