Kotlin Android – Center Align text in TextView
To center align text in TextView in Kotlin Android, set android:textAlignment attribute with the value “center” in layout file, or programmatically set the textAlignment property of the TextView object with View.TEXT_ALIGNMENT_CENTER in activity file.
The following code snippet demonstrates to center align text in TextView.
<TextView ... android:textAlignment="center" />
or
var textView = findViewById<TextView>(R.id.textView) textView.textAlignment = View.TEXT_ALIGNMENT_CENTER
Example Android Application
Let us create an Android Application with TextView in LinearLayout.
Refer – Android TextView Tutorial to create an Android Application with a TextView view object.
Center Align Text via Layout File
To center align the text in this Textview through layout file, set the android:textAlignment attribute for the TextView to “center”, as shown in the following activity_main.xml layout file.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <TextView android:id="@+id/textView" android:layout_width="wrap_content" android:layout_height="wrap_content" android:textAlignment="center" android:paddingTop="20dp" android:textSize="20sp" android:text="The quick brown fox jumps over the lazy dog. The quick brown fox jumps over the lazy dog." /> </LinearLayout>
Keep content of MainActivity.kt file as is.
Run the Android Application and TextView with the center aligned text appears as shown in the following screenshot.
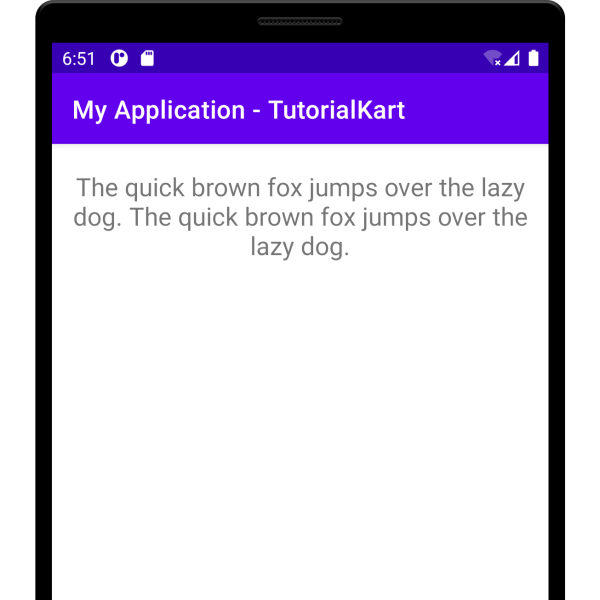
Center Align Text Programmatically in Kotlin Activity File
To center align the text in this Textview through Kotlin Activity file programmatically, get the reference to the TextView using id in layout file and set the textAlignment
property of the TextView object with View.TEXT_ALIGNMENT_CENTER
.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <TextView android:id="@+id/textView" android:layout_width="wrap_content" android:layout_height="wrap_content" android:paddingTop="20dp" android:textSize="20sp" android:text="The quick brown fox jumps over the lazy dog. The quick brown fox jumps over the lazy dog." /> </LinearLayout>
MainActivity.kt
package com.example.myapplication import androidx.appcompat.app.AppCompatActivity import android.os.Bundle import android.view.View import android.widget.TextView class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) var textView = findViewById<TextView>(R.id.textView) textView.textAlignment = View.TEXT_ALIGNMENT_CENTER } }
Run the Android Application and TextView with the center aligned text appears as shown in the following screenshot.
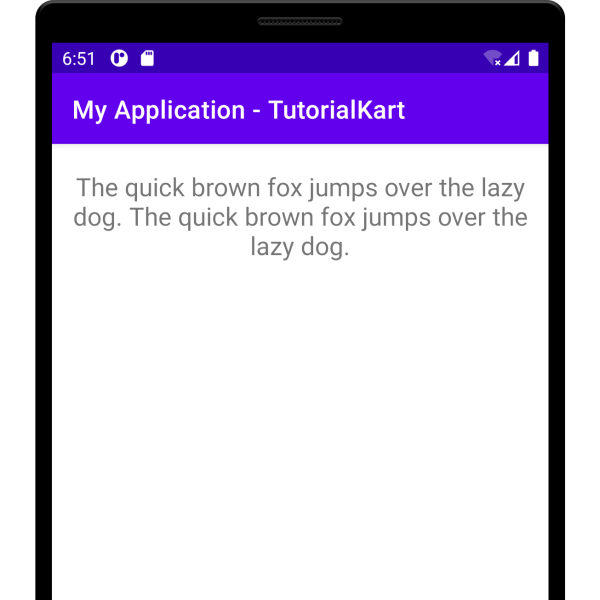
Conclusion
In this Kotlin Android Tutorial, we learned how to center align text in TextView in Android Application.