Kotlin Android – Change Background Color of Action Bar
To change background color of Action Bar in Kotlin Android, set the colorPrimary in themes.xml, with a required color. We can also dynamically change the background color of Action Bar programmatically by setting background drawable for support action bar with the required color drawable.
In this tutorial, we will go through the two processes: via themes.xml and via Kotlin file, to change the background color of Action Bar in Android Application.
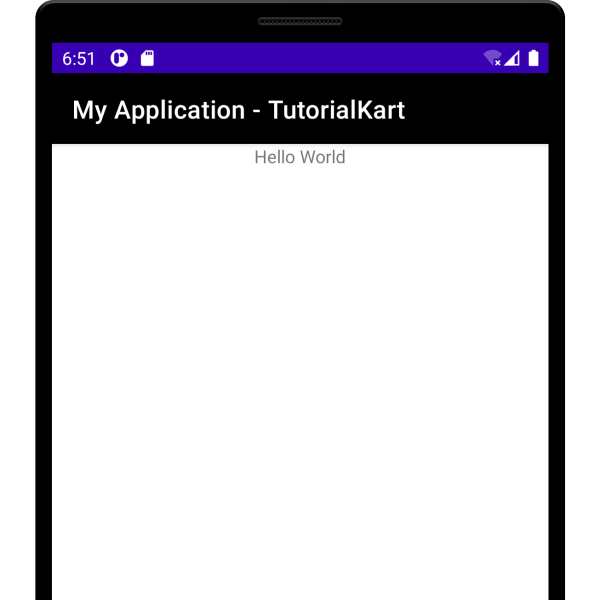
Change Background of Action Bar via themes.xml
The following is a step by step process to change the background color of Action Bar in Android Application.
Step 1 – Open themes.xml
1. Click on Resource Manager present on the left side of the Android Studio window.
2. In this Resource Manager window, click on Style tab.
3. Double click on the theme for our application. In this case, Theme.MyApplication.
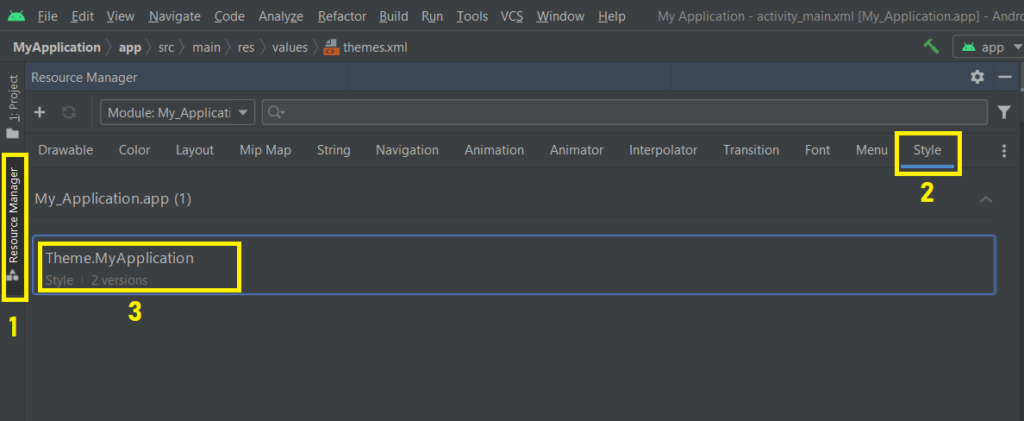
Theme items present for this theme will be displayed as shown in the following screenshot.
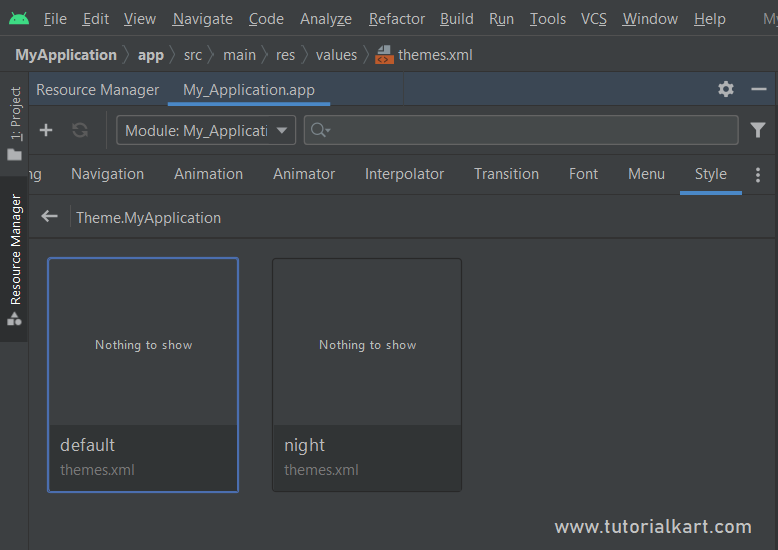
Double click on the default theme.
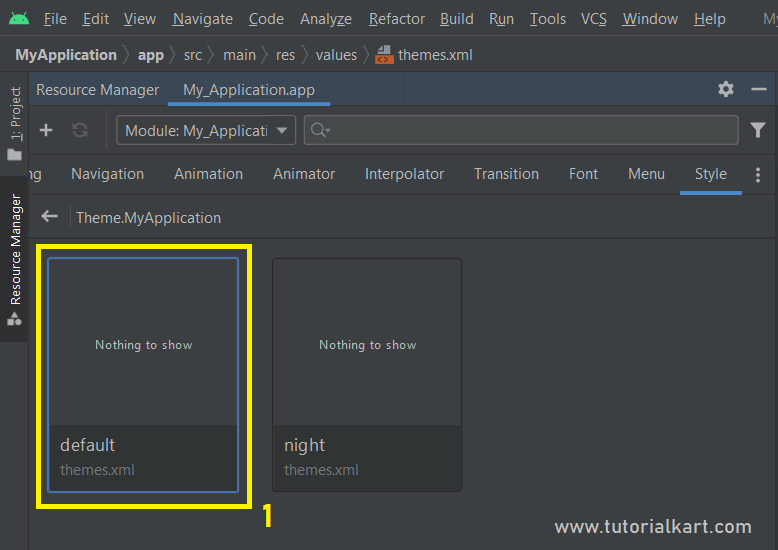
The contents of this style file, themes.xml, will be displayed in the editor.
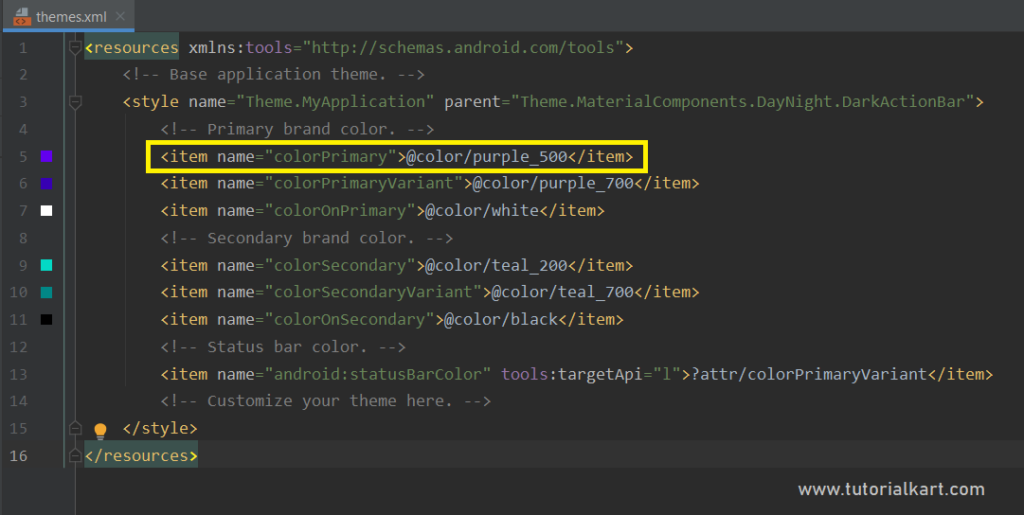
Step 2 – Change colors
Now, we have to change color value for the item with the attribute colorPrimary.
Let us assign black color to this item.
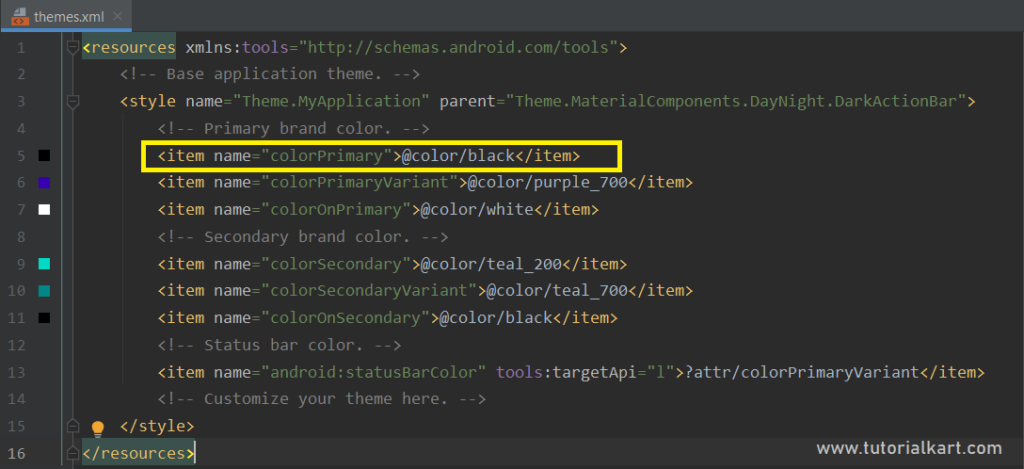
Step 3 – Run
Now, let us run this Android Application and observe the background color of Action Bar.
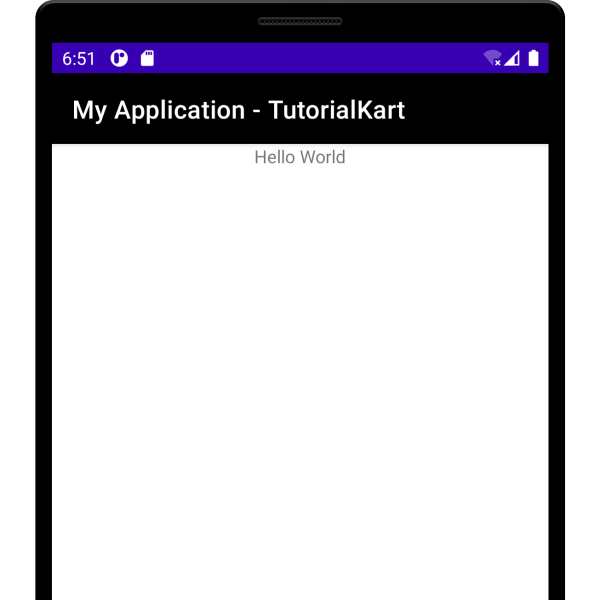
The background color of our Android Application is changed to the set color.
Change Background of Action Bar via Kotlin Activity File
To change the background color of Action Bar programmatically or dynamically, set background drawable for support action bar with the required Color drawable.
In the following example, we set the background color for Action Bar with the color represented by hex value “#146775” in RGB format.
MainActivity.kt
package com.example.myapplication import android.graphics.Color import android.graphics.drawable.ColorDrawable import android.os.Bundle import androidx.appcompat.app.AppCompatActivity class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) supportActionBar!!.setBackgroundDrawable(ColorDrawable(Color.parseColor("#146775"))) } }
Run the Android Application, and the background color of Action Bar will be set to the color "#146775"
, as shown in the following screenshot.
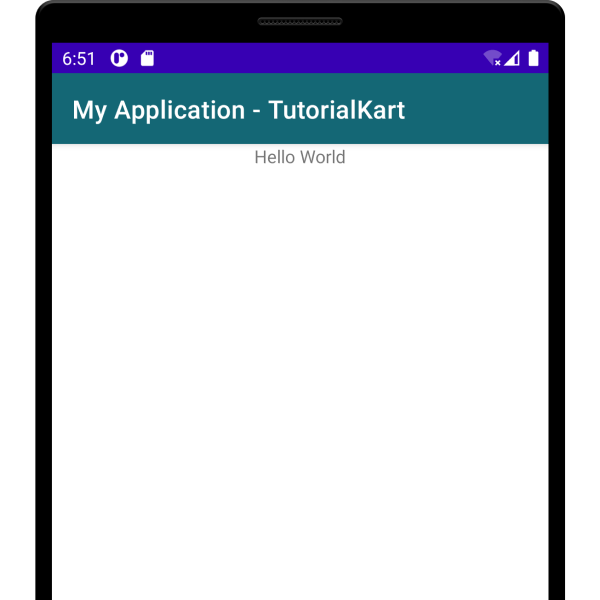
Conclusion
In this Kotlin Android Tutorial, we learned how to change the background color of Floating Action Button (FAB) widget via layout file or programmatically in Kotlin Activity file, with examples.