Kotlin Android – Change Icon Color of Floating Action Button
To change icon color of Floating Action Button in Kotlin Android we have to set the tint attribute (in layout file) or imageTintList parameter (in Kotlin program) of FAB with the required color.
To change the icon color of Floating Action Button in layout file, set the app:tint attribute with the required color value as shown in the following code snippet.
<com.google.android.material.floatingactionbutton.FloatingActionButton
...
app:tint="#EEE"
/>
To change the icon color of Floating Action Button dynamically or programmatically in Kotlin activity file, set the imageTintList parameter of the FAB with the required color value as shown in the following code snippet.
val fab = findViewById<FloatingActionButton>(R.id.floatingActionButton)
fab.imageTintList= ColorStateList.valueOf(Color.rgb(255, 50, 50))
Example – Change Icon Color of FAB via Layout File
Create an Android Application with Empty Activity and modify the activity_main.xml with the following code.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@+id/floatingActionButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:clickable="true"
app:backgroundTint="#EEE"
app:tint="#E91E63"
android:layout_marginTop="20dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.498"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:srcCompat="?android:attr/actionModeWebSearchDrawable" />
</androidx.constraintlayout.widget.ConstraintLayout>
Run this Android Application, and we would get the output as shown in the following screenshot, with the color of icon in Floating Action Button (FAB) changed to the given color value of #E91E63
.
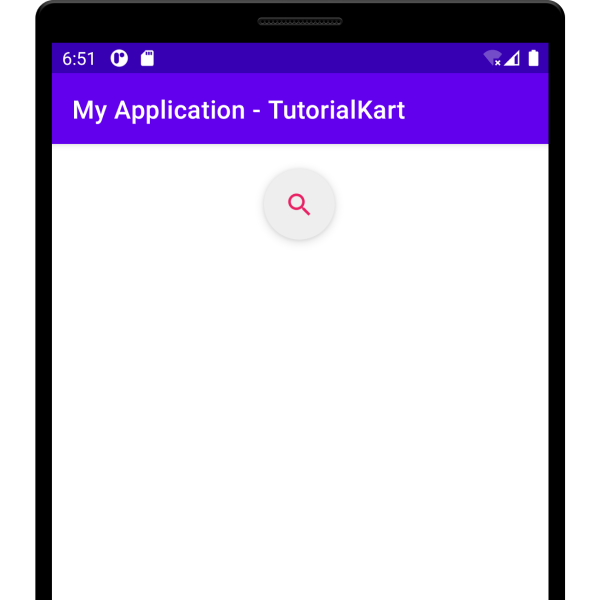
Example – Change Icon Color of FAB Programmatically
Create an Android Application with Empty Activity and modify the activity_main.xml and MainActivity.kt with the following code.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@+id/floatingActionButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:clickable="true"
app:backgroundTint="#EEE"
android:layout_marginTop="20dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.498"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:srcCompat="?android:attr/actionModeWebSearchDrawable" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.kt
package com.example.myapplication
import android.content.res.ColorStateList
import android.graphics.Color
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import com.google.android.material.floatingactionbutton.FloatingActionButton
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val fab = findViewById<FloatingActionButton>(R.id.floatingActionButton)
fab.imageTintList = ColorStateList.valueOf(Color.rgb(255, 50, 50))
}
}
Run this Android Application, and we would get the output as shown in the following screenshot, with the icon color of Floating Action Button (FAB) changed to the given color value of Color.rgb(255, 50, 50)
.
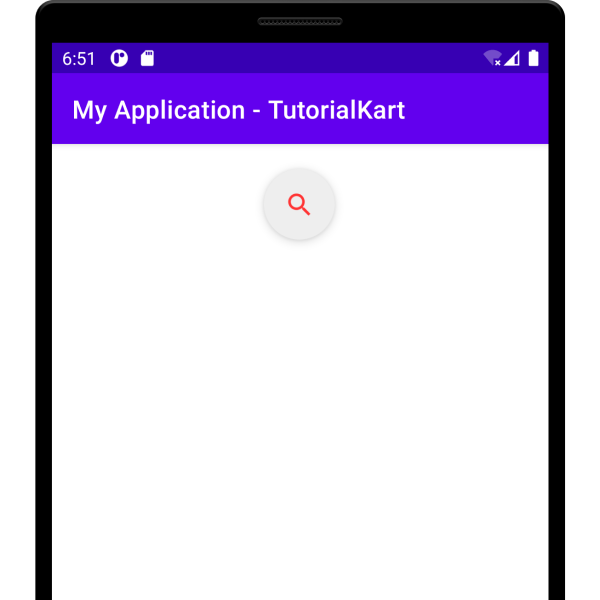
Conclusion
In this Kotlin Android Tutorial, we learned how to change the background color of Floating Action Button (FAB) widget via layout file or programmatically in Kotlin Activity file, with examples.