Kotlin Android – Set Tint Color & Tint Mode for ImageView
To set tint color and tint mode for ImageView in Kotlin Android, set app:tint and android:tintMode attributes of ImageView in layout file with required color and tint mode respectively.
The following code snippet demonstrates to set specific tint color and tint mode for ImageView.
<ImageView
...
app:tint="#0ff"
android:tintMode="multiply"
/>
Example Android Application
Let us create an Android Application with ImageView.
Refer Kotlin Android – ImageView Example to create an Android Application with just an ImageView in LinearLayout.
The project structure in Android mode is
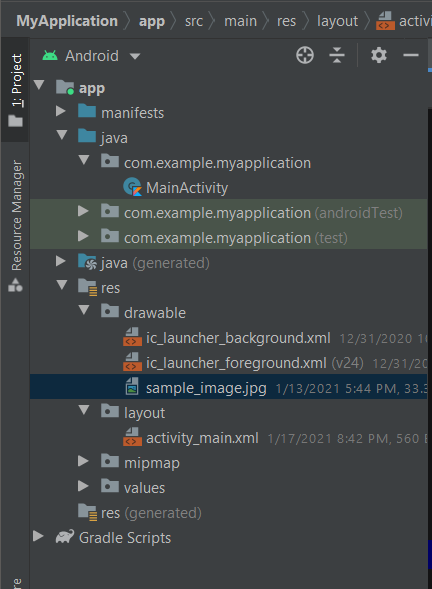
Without any tint applied, the image appears as shown in the following screenshot.
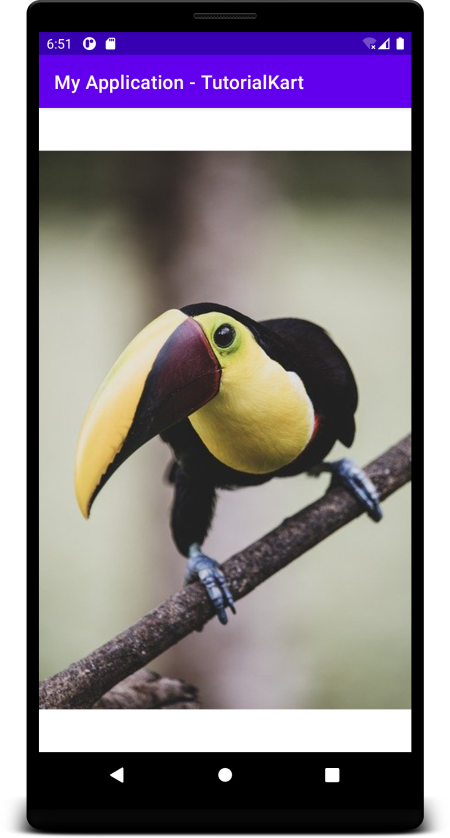
Set Tint Color
Open activity_main.xml file and set the attribute app:tint for ImageView with the a specific color as shown in the following.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:orientation="vertical">
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:tint="#0ff"
app:srcCompat="@drawable/sample_image" />
</LinearLayout>
Run the Android Application
Now, let us run the Android Application, and see how the tint color affected the ImageView.
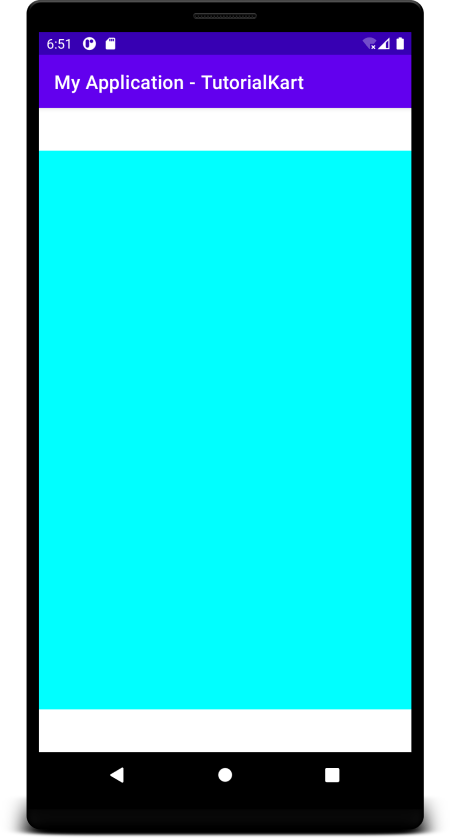
The tint color is shown for ImageView but not the image. The reason is, the default value of tint mode is src_atop. Let us specify another value for tint mode using android:tintMode attribute.
In the following activity_main.xml file, we have set the tint mode to multiply.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:orientation="vertical">
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:tint="#0ff"
android:tintMode="multiply"
app:srcCompat="@drawable/sample_image" />
</LinearLayout>
Now, run the application. The tint will be applied based on the tint mode multiply.
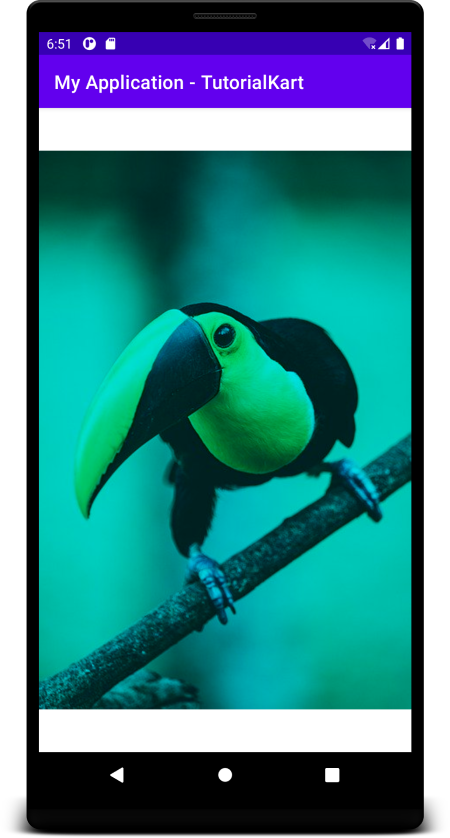
The following list specifies the possible values for tint mode.
- add
- multiply
- screen
- src_atop
- src_in
- src_over
Change the values for tint and tint mode and observe the output.
Conclusion
In this Kotlin Android Tutorial, we learned how to set specific tint color and tint mode for ImageView in Android Application.