Android LinearLayout – Center Align
To center align LinearLayout, assign android:gravity attribute of this LinearLayout with the value “center”.
</>
Copy
android:gravity="center"
Let us create an Android application with LinearLayout containing two Button widgets as children. We shall center align these children using gravity attribute.
activity_main.xml
</>
Copy
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:orientation="vertical">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="10sp"
android:text="Reset" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="10sp"
android:text="Submit" />
</LinearLayout>
MainActivity.kt
</>
Copy
package com.example.myapplication
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
}
}
Run this application and you would get the output as shown in the following screenshot.
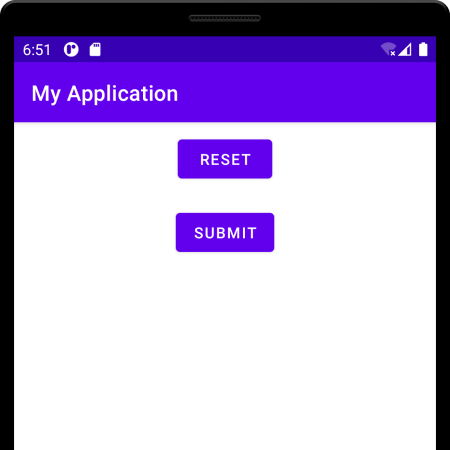
If the orientation of LinearLayout is horizontal, then the center aligned LinearLayout would look as shown in the following screenshot.
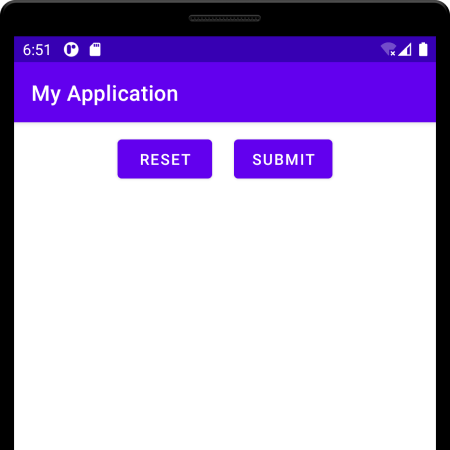
Conclusion
In this Kotlin Android Tutorial, we learned how to center align LinearLayout.