Kotlin Android – TextView Shadow Effect
To get shadow for TextView in Android, set android:elevation attribute, with required amount of elevation from the base level, for the TextView widget in layout XML file. For elevation to be effective, we should set background for the TextView.
<TextView
...
android:background="#FFF"
android:elevation="10dp" />
TextViews with different elevation values: 10dp, 20dp and 30dp respectively from top to bottom, would look like as shown in the following screenshot.
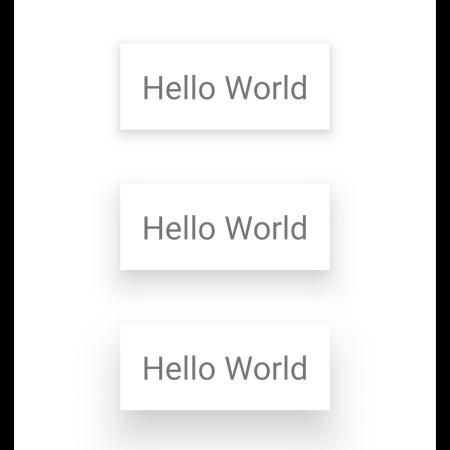
Example
In this example, we will create an Android Application with three TextView widgets, each of them having a different elevation.
Create an Android Application with an Empty Activity.
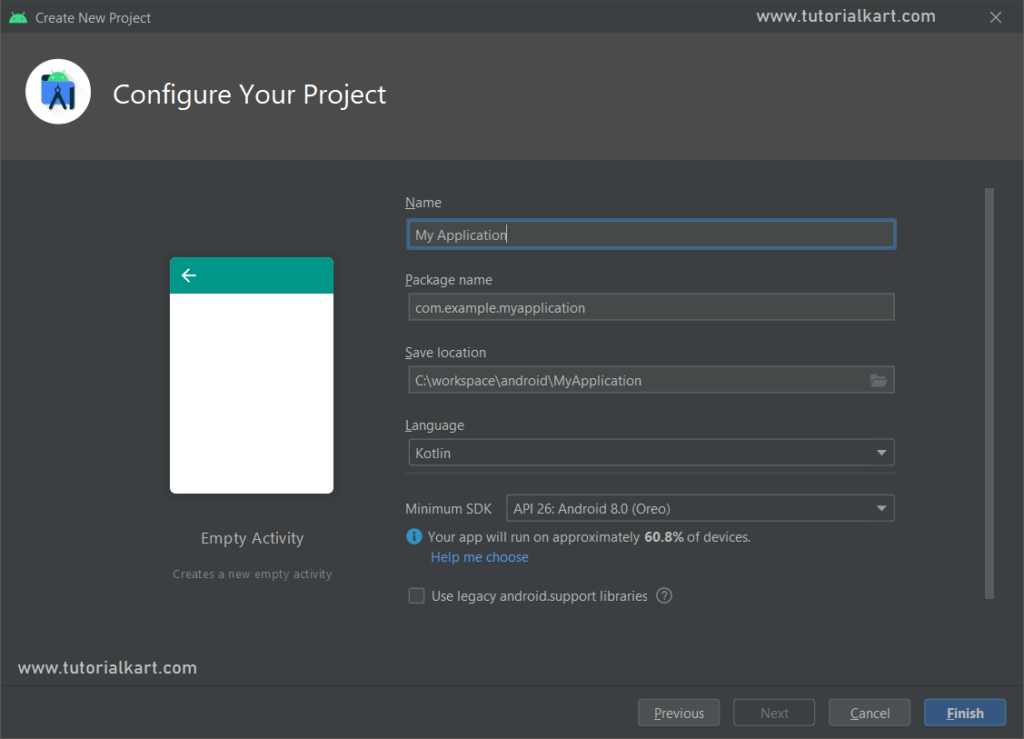
In the main activity layout file, create three TextView widgets with elevation values 10dp, 20dp and 30dp respectively. All of these TextView widgets are given with background of “#FFF”.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:gravity="center">
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textIsSelectable="true"
android:textSize="30sp"
android:padding="20sp"
android:background="#FFF"
android:elevation="10dp"
android:layout_marginBottom="50dp"
android:text="Hello World" />
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textIsSelectable="true"
android:textSize="30sp"
android:padding="20sp"
android:background="#FFF"
android:elevation="20dp"
android:layout_marginBottom="50dp"
android:text="Hello World" />
<TextView
android:id="@+id/textView3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textIsSelectable="true"
android:textSize="30sp"
android:padding="20sp"
android:background="#FFF"
android:elevation="30dp"
android:text="Hello World" />
</LinearLayout>
No changes are required in MainActivity.kt file.
MainActivity.kt
package com.example.myapplication
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
}
}
Run this Android Application and you should get the TextView widgets displayed with elevation or say shadow.
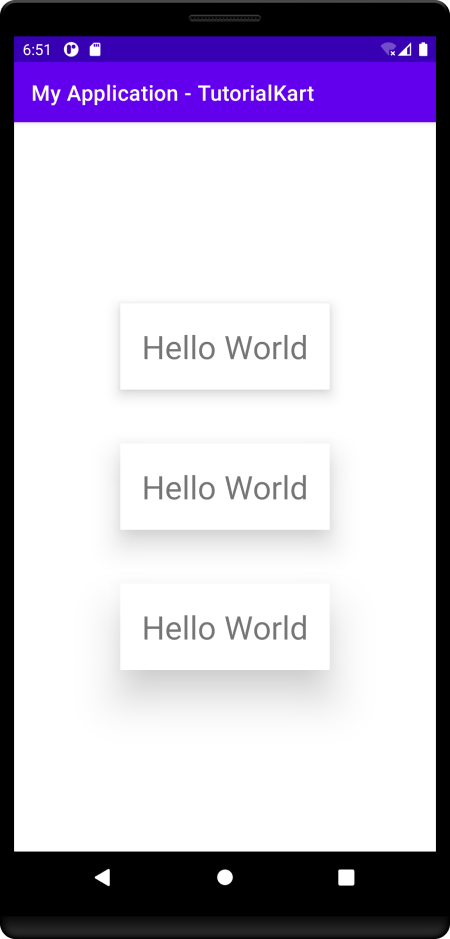
Conclusion
In this Kotlin Android Tutorial, we learned how to get shadow effect for TextView in Android.