Constants in Swift
To define constants in Swift, we can use let
keyword. When we define a variable using let keyword, the variable becomes immutable, meaning the value initialised can never be modified.
If we try to modify the value of a constant, Swift raises Compile time error “Cannot assign to value”.
In this tutorial, we will learn how to define a constant in Swift using let keyword.
Example
In the following program, we will define a constant PI
using let
keyword.
main.swift
let PI = 3.14
print(PI)
We can use these constants in other expressions. For demonstration, let us write a program to with function that calculates the area of circle. This function makes use the PI
constant we defined in the above program.
main.swift
let PI = 3.14
func circleArea (radius: Double)-> Double {
return PI*radius*radius
}
print("Area of cirle with radius=3 is \(circleArea(radius: 3.0))")
Output
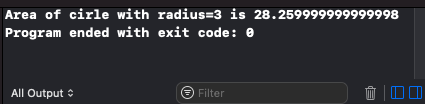
Conclusion
In this Swift Tutorial, we learned how to define a constant in Swift using let
keyword.